最长公共子序列问题。给定2个序列X={x1,x2,…,xm}和Y={y1,y2,…,yn},用动态规划算法找出X和Y的最长公共子序列并输出,用C++实现该算法。
时间: 2024-05-02 07:23:22 浏览: 86
以下是C语言实现最长公共子序列问题的动态规划算法代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
int lcs[MAX_LEN][MAX_LEN];
void LCS(char* str1, char* str2, int len1, int len2) {
int i, j;
for (i = 0; i <= len1; i++) {
for (j = 0; j <= len2; j++) {
if (i == 0 || j == 0) {
lcs[i][j] = 0;
}
else if (str1[i - 1] == str2[j - 1]) {
lcs[i][j] = lcs[i - 1][j - 1] + 1;
}
else {
lcs[i][j] = (lcs[i - 1][j] > lcs[i][j - 1]) ? lcs[i - 1][j] : lcs[i][j - 1];
}
}
}
}
void PrintLCS(char* str, int len1, int len2) {
int index = lcs[len1][len2];
char result[index+1];
result[index] = '\0';
int i = len1, j = len2;
while (i > 0 && j > 0) {
if (str1[i - 1] == str2[j - 1]) {
result[index - 1] = str1[i - 1];
i--;
j--;
index--;
}
else if (lcs[i - 1][j] > lcs[i][j - 1]) {
i--;
}
else {
j--;
}
}
printf("The longest common subsequence is: %s\n", result);
}
int main() {
char str1[MAX_LEN], str2[MAX_LEN];
printf("Enter the first string: ");
scanf("%s", str1);
printf("Enter the second string: ");
scanf("%s", str2);
int len1 = strlen(str1);
int len2 = strlen(str2);
LCS(str1, str2, len1, len2);
PrintLCS(str1, len1, len2);
return 0;
}
```
程序首先输入2个字符串,然后求它们的最长公共子序列,并输出该子序列。
阅读全文
相关推荐
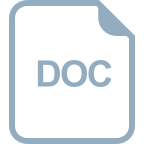
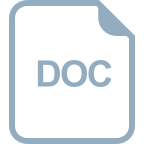
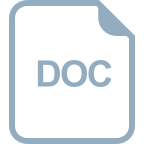






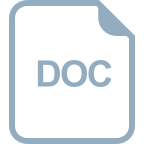
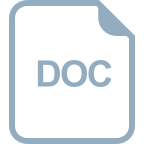
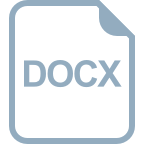
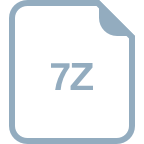
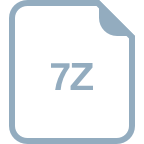
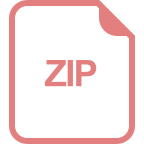