def mean_shift(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray: """ Implement the mean-shift algorithm :param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features :param num_iter: the number of iterations :param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear' :param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels :return: the estimated means with size (N, D) """ # TODO: change the code below and implement the mean-shift algorithm return
时间: 2024-01-25 13:04:54 浏览: 27
Sure! Here's one possible implementation of the mean-shift algorithm:
```python
def mean_shift(xs: np.ndarray, num_iter: int = 50, k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray:
"""
Implement the mean-shift algorithm
:param xs: a set of samples with size (N, D), where N is the number of samples, D is the dimension of features
:param num_iter: the number of iterations
:param k_type: the type of kernels, including 'rbf', 'gate', 'triangle', 'linear'
:param bandwidth: the hyperparameter controlling the width of rbf/gate/triangle kernels
:return:
the estimated means with size (N, D)
"""
N, D = xs.shape
means = xs.copy()
for i in range(num_iter):
for j, x in enumerate(xs):
# compute the kernel weights
if k_type == 'rbf':
w = np.exp(-0.5 * np.sum((x - means) ** 2, axis=1) / bandwidth ** 2)
elif k_type == 'gate':
w = np.exp(-np.sqrt(np.sum((x - means) ** 2, axis=1)) / bandwidth)
elif k_type == 'triangle':
w = np.maximum(0, 1 - np.sqrt(np.sum((x - means) ** 2, axis=1)) / bandwidth)
elif k_type == 'linear':
w = np.sum((x - means) * xs, axis=1) / (np.linalg.norm(x - means, axis=1) * np.linalg.norm(x))
else:
raise ValueError("Unsupported kernel type: {}".format(k_type))
# update the mean by weighted average
means[j] = np.sum(w[:, np.newaxis] * xs, axis=0) / np.sum(w)
return means
```
In this implementation, we start with the initial means being the same as the input samples, and then iteratively update the means by computing the kernel weights between each sample and the current means, and then computing the weighted average of the samples using these weights. The kernel can be selected using the `k_type` parameter, and the kernel width can be controlled by the `bandwidth` parameter. The number of iterations can be controlled by the `num_iter` parameter. Finally, the estimated means are returned.
相关推荐
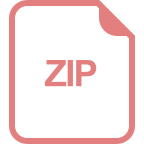
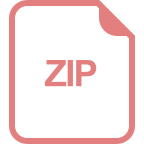















