5. java编写克鲁斯卡尔算法构造最小生成树
时间: 2024-02-05 18:36:38 浏览: 32
克鲁斯卡尔算法是一种求解最小生成树的经典算法,其基本思想是贪心算法。以下是使用Java实现克鲁斯卡尔算法构造最小生成树的代码:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
class Graph {
private int[] parent;
private int[] rank;
public Graph(int n) {
parent = new int[n];
rank = new int[n];
for (int i = 0; i < n; i++) {
parent[i] = i;
rank[i] = 0;
}
}
private int find(int x) {
if (parent[x] != x) {
parent[x] = find(parent[x]);
}
return parent[x];
}
private void union(int x, int y) {
int xRoot = find(x);
int yRoot = find(y);
if (rank[xRoot] < rank[yRoot]) {
parent[xRoot] = yRoot;
} else if (rank[xRoot] > rank[yRoot]) {
parent[yRoot] = xRoot;
} else {
parent[xRoot] = yRoot;
rank[yRoot]++;
}
}
public List<Edge> kruskalMST(List<Edge> edges) {
List<Edge> result = new ArrayList<>();
Collections.sort(edges, Comparator.comparingInt(e -> e.weight));
for (Edge edge : edges) {
int u = edge.u;
int v = edge.v;
int w = edge.weight;
if (find(u) != find(v)) {
union(u, v);
result.add(edge);
}
}
return result;
}
}
class Edge {
int u;
int v;
int weight;
public Edge(int u, int v, int weight) {
this.u = u;
this.v = v;
this.weight = weight;
}
}
public class KruskalMST {
public static void main(String[] args) {
int n = 5;
List<Edge> edges = new ArrayList<>();
edges.add(new Edge(0, 1, 2));
edges.add(new Edge(0, 3, 6));
edges.add(new Edge(1, 2, 3));
edges.add(new Edge(1, 3, 8));
edges.add(new Edge(1, 4, 5));
edges.add(new Edge(2, 4, 7));
edges.add(new Edge(3, 4, 9));
Graph graph = new Graph(n);
List<Edge> result = graph.kruskalMST(edges);
for (Edge edge : result) {
System.out.println(edge.u + " - " + edge.v + ": " + edge.weight);
}
}
}
```
在上面的代码中,我们首先定义了一个Graph类,其中包含了find和union两个函数,分别用于查找节点的根节点和合并两个不同的集合。然后,我们定义了一个Edge类,用于表示图中的边。最后,在main函数中,我们定义了一个包含边的列表,然后使用Graph类的kruskalMST函数求解最小生成树,并打印结果。
相关推荐
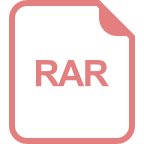














