c++如何计算从100到500之间的素数的个数?
时间: 2024-12-15 15:14:38 浏览: 3
在C++中,我们可以使用埃拉托斯特尼筛法(Sieve of Eratosthenes)来计算100到500之间的素数个数。以下是简单的步骤:
1. 创建一个布尔数组isPrime,大小为501,初始化所有元素为true,因为默认认为每个数字都是质数。
2. 从2开始(第一个质数),遍历数组,将2的倍数标记为非质数(isPrime[2*i] = false)。
3. 然后找到下一个未被标记为非质数的数字(即下一个素数),将其赋值给变量`currentPrime`(当前正在检查的数)。
4. 更新`currentPrime`的值,并继续遍历,每次增加2,直到`currentPrime`大于500为止。
5. 遍历结束后,isPrime数组中仍为true的索引对应的数字就是素数,统计真值的数量即为素数个数。
下面是一个示例C++代码片段:
```cpp
#include <iostream>
using namespace std;
int countPrimes(int n) {
bool isPrime[n+1];
memset(isPrime, true, sizeof(isPrime));
for (int p=2; p*p<=n; p++) {
if (isPrime[p]) {
for (int i=p*2; i<=n; i += p)
isPrime[i] = false;
}
}
int primeCount = 0;
for (int i=2; i<=n; ++i)
if (isPrime[i])
primeCount++;
return primeCount;
}
int main() {
int start = 100;
int end = 500;
int result = countPrimes(end);
cout << "Number of primes between " << start << " and " << end << " is: " << result << endl;
return 0;
}
```
阅读全文
相关推荐
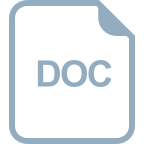
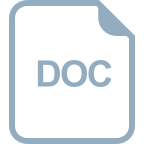
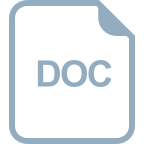















