python写一个简单的贪吃蛇
时间: 2024-01-07 18:02:29 浏览: 67
好的,下面是一个简单的贪吃蛇游戏的 Python 代码:
```python
import pygame
import random
# 游戏初始化
pygame.init()
# 游戏窗口大小
win_width = 500
win_height = 500
win = pygame.display.set_mode((win_width, win_height))
pygame.display.set_caption("贪吃蛇")
# 颜色定义
black = (0, 0, 0)
white = (255, 255, 255)
green = (0, 255, 0)
red = (255, 0, 0)
# 蛇的初始位置和大小
snake_block = 10
snake_list = []
snake_length = 1
# 蛇的移动速度
speed = 15
# 随机食物的位置
food_x = round(random.randrange(0, win_width - snake_block) / 10.0) * 10.0
food_y = round(random.randrange(0, win_height - snake_block) / 10.0) * 10.0
# 游戏结束的标志
game_over = False
# 设置字体
font_style = pygame.font.SysFont(None, 50)
# 显示分数
def show_score(score):
score_font = font_style.render("得分: " + str(score), True, white)
win.blit(score_font, [0, 0])
# 画蛇
def draw_snake(snake_block, snake_list):
for x in snake_list:
pygame.draw.rect(win, green, [x[0], x[1], snake_block, snake_block])
# 显示消息
def message(msg, color):
msg_font = font_style.render(msg, True, color)
win.blit(msg_font, [win_width / 6, win_height / 3])
# 游戏循环
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
# 蛇的移动
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
x_change = -snake_block
y_change = 0
elif event.key == pygame.K_RIGHT:
x_change = snake_block
y_change = 0
elif event.key == pygame.K_UP:
x_change = 0
y_change = -snake_block
elif event.key == pygame.K_DOWN:
x_change = 0
y_change = snake_block
# 蛇头的位置
snake_head = []
snake_head.append(food_x)
snake_head.append(food_y)
snake_list.append(snake_head)
# 当蛇的长度超过设定值时,删除蛇尾
if len(snake_list) > snake_length:
del snake_list[0]
# 当蛇头和食物重合时,重新生成食物,并且蛇的长度加1
if food_x == snake_head[0] and food_y == snake_head[1]:
food_x = round(random.randrange(0, win_width - snake_block) / 10.0) * 10.0
food_y = round(random.randrange(0, win_height - snake_block) / 10.0) * 10.0
snake_length += 1
# 当蛇头碰撞到墙壁或者碰到自己的身体时,游戏结束
if snake_head[0] >= win_width or snake_head[0] < 0 or snake_head[1] >= win_height or snake_head[1] < 0:
game_over = True
for x in snake_list[:-1]:
if x == snake_head:
game_over = True
# 游戏窗口背景颜色
win.fill(black)
# 画食物和蛇
pygame.draw.rect(win, red, [food_x, food_y, snake_block, snake_block])
draw_snake(snake_block, snake_list)
# 显示分数
show_score(snake_length - 1)
# 更新游戏窗口
pygame.display.update()
# 蛇的移动
food_x += x_change
food_y += y_change
# 控制游戏速度
clock = pygame.time.Clock()
clock.tick(speed)
# 游戏结束时显示分数
message("游戏结束", red)
pygame.display.update()
# 游戏结束后等待3秒钟,然后退出游戏
pygame.time.wait(3000)
# 退出游戏
pygame.quit()
quit()
```
注意:本代码仅为一个简单的贪吃蛇游戏,还有很多需要改进的地方,比如蛇的移动方式、游戏难度等。
阅读全文
相关推荐
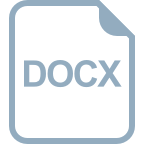
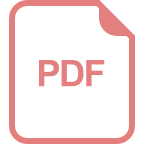













