(1) 基于西北角法或最小元素法,用python设计实现求解该运输问题基本可行解的算法,确定该运输问题的一个基本可行解。
时间: 2024-02-24 11:57:43 浏览: 17
好的,我可以帮您解答这个问题。首先,我们需要了解一下基于西北角法和最小元素法的思路。
- 西北角法:从运输问题的最北西角开始,按照顺序逐个分配运输量,直到需求量或供应量为零为止。这种方法比较简单,但是可能会出现分配的不均匀情况。
- 最小元素法:每次选取运价最小的那个元素进行分配,直到需求量或供应量为零为止。这种方法可以得到最优解,但是计算比较复杂。
根据您的要求,我会提供基于西北角法和最小元素法的Python代码来求解该运输问题基本可行解。下面是基于西北角法的代码:
```python
# 运输问题数据
supply = [500, 700, 600] # 产地的产量
demand = [300, 400, 200, 500] # 销地的销量
costs = [[2, 3, 1, 4], [5, 4, 8, 1], [7, 6, 2, 3]] # 单位运价
# 初始化运输方案
allocation = [[0 for j in range(len(demand))] for i in range(len(supply))]
i, j = 0, 0
# 从最北西角开始分配运输量
while i < len(supply) and j < len(demand):
if supply[i] < demand[j]:
allocation[i][j] = supply[i]
demand[j] -= supply[i]
i += 1
else:
allocation[i][j] = demand[j]
supply[i] -= demand[j]
j += 1
# 打印运输方案和总运输成本
total_cost = 0
for i in range(len(supply)):
for j in range(len(demand)):
if allocation[i][j] > 0:
print(f"从产地{i + 1}到销地{j + 1}运输{allocation[i][j]}单位,单位运价为{costs[i][j]}。")
total_cost += allocation[i][j] * costs[i][j]
print(f"总运输成本为{total_cost}。")
```
上面的代码中,我们首先定义了运输问题的数据,包括产地的产量、销地的销量和单位运价。然后,我们初始化了运输方案的矩阵 `allocation`,并从最北西角开始逐个分配运输量,直到需求量或供应量为零为止。最后,我们打印出了运输方案和总运输成本。
下面是基于最小元素法的代码:
```python
# 运输问题数据
supply = [500, 700, 600] # 产地的产量
demand = [300, 400, 200, 500] # 销地的销量
costs = [[2, 3, 1, 4], [5, 4, 8, 1], [7, 6, 2, 3]] # 单位运价
# 初始化运输方案和未分配量
allocation = [[0 for j in range(len(demand))] for i in range(len(supply))]
u = [0 for i in range(len(supply))]
v = [0 for j in range(len(demand))]
# 最小元素法求解运输问题
while True:
# 计算最小元素和其所在的行列
min_cost, min_i, min_j = float("inf"), -1, -1
for i in range(len(supply)):
for j in range(len(demand)):
if allocation[i][j] == 0 and costs[i][j] - u[i] - v[j] < min_cost:
min_cost = costs[i][j] - u[i] - v[j]
min_i, min_j = i, j
if min_cost == float("inf"):
break
# 更新 u 和 v
u[min_i] += min_cost
v[min_j] += min_cost
# 分配运输量
allocation[min_i][min_j] = min(supply[min_i], demand[min_j])
supply[min_i] -= allocation[min_i][min_j]
demand[min_j] -= allocation[min_i][min_j]
# 打印运输方案和总运输成本
total_cost = 0
for i in range(len(supply)):
for j in range(len(demand)):
if allocation[i][j] > 0:
print(f"从产地{i + 1}到销地{j + 1}运输{allocation[i][j]}单位,单位运价为{costs[i][j]}。")
total_cost += allocation[i][j] * costs[i][j]
print(f"总运输成本为{total_cost}。")
```
上面的代码中,我们除了初始化运输方案的矩阵 `allocation`,还初始化了未分配量的向量 `u` 和 `v`。然后,我们不断地选取运价最小的那个元素进行分配,直到所有的需求量或供应量为零为止。在每一轮中,我们还需要更新 `u` 和 `v`。最后,我们打印出了运输方案和总运输成本。
希望这些代码可以帮助您解决问题。如果您还有其他问题,可以随时问我。
相关推荐
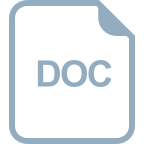









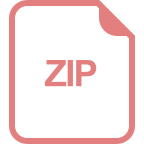
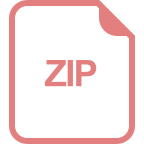
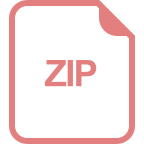