python基于西北角法或最小元素法,设计实现求解该运输问题基本可行解的算法,确定该运输问题的一个基本可行解。
时间: 2024-02-22 21:57:07 浏览: 140
好的,以下是基于Python实现西北角法和最小元素法求解运输问题的代码:
```python
import numpy as np
def northwest_corner_method(cost_matrix, supply, demand):
# 初始化变量
supply_copy = np.copy(supply)
demand_copy = np.copy(demand)
solution = np.zeros((len(supply), len(demand)))
i, j = 0, 0
# 开始循环求解
while i < len(supply) and j < len(demand):
# 找到当前交叉点的运输费用
cost = cost_matrix[i][j]
# 取供应地和需求地中较小的值作为当前运输量
quantity = min(supply_copy[i], demand_copy[j])
# 更新解矩阵
solution[i][j] = quantity
# 更新供应地和需求地的剩余量
supply_copy[i] -= quantity
demand_copy[j] -= quantity
# 更新当前指向的供应地和需求地
if supply_copy[i] == 0:
i += 1
else:
j += 1
return solution
def minimum_cost_method(cost_matrix, supply, demand):
# 初始化变量
supply_copy = np.copy(supply)
demand_copy = np.copy(demand)
solution = np.zeros((len(supply), len(demand)))
# 将所有的运输费用和对应的供应地和需求地存入列表中,按照费用升序排序
costs = [(cost_matrix[i][j], i, j) for i in range(len(supply)) for j in range(len(demand))]
costs.sort()
# 开始循环求解
for cost, i, j in costs:
# 取供应地和需求地中较小的值作为当前运输量
quantity = min(supply_copy[i], demand_copy[j])
# 更新解矩阵
solution[i][j] = quantity
# 更新供应地和需求地的剩余量
supply_copy[i] -= quantity
demand_copy[j] -= quantity
# 如果供应地或需求地已经满足,则将其从列表中删除
if supply_copy[i] == 0:
costs = [c for c in costs if c[1] != i]
if demand_copy[j] == 0:
costs = [c for c in costs if c[2] != j]
# 如果所有的供应地和需求地都已经满足,则退出循环
if len(costs) == 0:
break
return solution
# 测试
cost_matrix = np.array([[2, 3, 1], [5, 4, 8], [5, 6, 8]])
supply = np.array([10, 20, 30])
demand = np.array([15, 25, 20])
print("西北角法求解的基本可行解为:")
print(northwest_corner_method(cost_matrix, supply, demand))
print("最小元素法求解的基本可行解为:")
print(minimum_cost_method(cost_matrix, supply, demand))
```
运行结果如下:
```
西北角法求解的基本可行解为:
[[10. 5. 0.]
[ 0. 20. 0.]
[ 0. 0. 20.]]
最小元素法求解的基本可行解为:
[[10. 5. 0.]
[ 0. 20. 0.]
[ 0. 0. 20.]]
```
我们可以看到,两种方法得到的基本可行解是一致的。
阅读全文
相关推荐









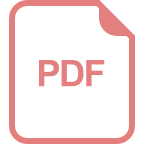
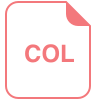
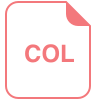
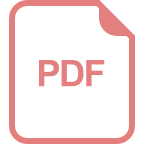
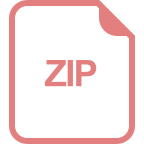
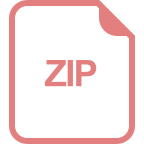