任意给的直线和圆的方程,求它们公切圆的方程c语言
时间: 2024-03-22 11:39:31 浏览: 37
假设给出的直线方程为 ax + by + c = 0,圆的方程为 (x - p)^2 + (y - q)^2 = r^2,其中 (p,q) 为圆心坐标,r 为半径。
则公切圆的方程为 (ax + by + c)^2 = r^2*(a^2 + b^2)。
下面是用 C 语言实现的代码:
```c
#include <stdio.h>
#include <math.h>
void tangent_circle(double a, double b, double c, double p, double q, double r)
{
double d = fabs(a*p + b*q + c) / sqrt(a*a + b*b); // 直线到圆心的距离
if (d > r) // 直线与圆没有交点
{
printf("No tangent circle exists!\n");
return;
}
double x1, y1, x2, y2; // 交点坐标
if (a == 0) // 直线垂直于 y 轴
{
x1 = x2 = -c / b;
y1 = q + sqrt(r*r - d*d);
y2 = q - sqrt(r*r - d*d);
}
else if (b == 0) // 直线垂直于 x 轴
{
y1 = y2 = -c / a;
x1 = p + sqrt(r*r - d*d);
x2 = p - sqrt(r*r - d*d);
}
else // 直线不垂直于坐标轴
{
double k = -a / b;
double A = k*k + 1;
double B = 2*k*(c - b*q) - 2*p;
double C = p*p + (c - b*q)*(c - b*q) - r*r;
double delta = B*B - 4*A*C;
x1 = (-B + sqrt(delta)) / (2*A);
x2 = (-B - sqrt(delta)) / (2*A);
y1 = k*x1 + c / b;
y2 = k*x2 + c / b;
}
printf("The tangent circles are:\n");
printf("(x - %.2lf)^2 + (y - %.2lf)^2 = %.2lf^2\n", x1, y1, r);
printf("(x - %.2lf)^2 + (y - %.2lf)^2 = %.2lf^2\n", x2, y2, r);
}
int main()
{
double a, b, c, p, q, r;
printf("Please input the line equation ax + by + c = 0: ");
scanf("%lf %lf %lf", &a, &b, &c);
printf("Please input the circle equation (x - p)^2 + (y - q)^2 = r^2: ");
scanf("%lf %lf %lf", &p, &q, &r);
tangent_circle(a, b, c, p, q, r);
return 0;
}
```
该程序可以接受用户输入直线和圆的方程参数,计算出公切圆的方程并输出。注意,由于计算过程中存在开方运算,因此可能存在精度误差。
阅读全文
相关推荐
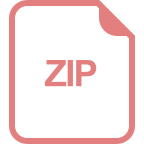



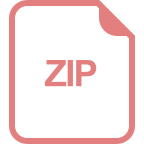
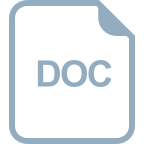
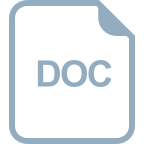
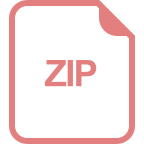
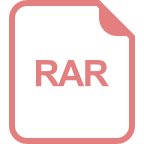

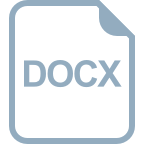
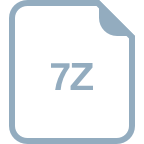
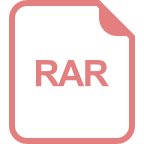
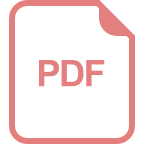