写一个unity按照给定法线方向生成半球体mesh的脚本
时间: 2023-05-23 07:00:55 浏览: 116
可以使用以下脚本实现:
```
using UnityEngine;
public class HalfSphereMeshGenerator : MonoBehaviour
{
[SerializeField] private int numLongitudeSections = 10;
[SerializeField] private int numLatitudeSections = 10;
[SerializeField] private float radius = 1f;
[SerializeField] private Vector3 upDirection = Vector3.up;
private void Start()
{
Mesh mesh = new Mesh();
Vector3[] vertices = new Vector3[numLongitudeSections * (numLatitudeSections + 1)];
int[] triangles = new int[numLongitudeSections * numLatitudeSections * 6];
for (int lat = 0; lat <= numLatitudeSections; lat++)
{
float theta = lat * Mathf.PI / numLatitudeSections;
float sinTheta = Mathf.Sin(theta);
float cosTheta = Mathf.Cos(theta);
for (int lon = 0; lon < numLongitudeSections; lon++)
{
float phi = lon * 2f * Mathf.PI / numLongitudeSections;
float sinPhi = Mathf.Sin(phi);
float cosPhi = Mathf.Cos(phi);
Vector3 direction = new Vector3(sinTheta * cosPhi, cosTheta, sinTheta * sinPhi);
Vector3 normal = Quaternion.FromToRotation(upDirection, direction) * upDirection;
vertices[lat * numLongitudeSections + lon] = normal * radius;
}
}
int index = 0;
for (int lat = 0; lat < numLatitudeSections; lat++)
{
for (int lon = 0; lon < numLongitudeSections; lon++)
{
int first = lat * numLongitudeSections + lon;
int second = first + numLongitudeSections;
triangles[index++] = first;
triangles[index++] = second + 1;
triangles[index++] = second;
triangles[index++] = first;
triangles[index++] = first + 1;
triangles[index++] = second + 1;
}
}
mesh.vertices = vertices;
mesh.triangles = triangles;
mesh.RecalculateNormals();
GetComponent<MeshFilter>().mesh = mesh;
}
}
```
这个脚本使用给定法线方向和半径,按照给定的经度和纬度划分数量,生成半球体的网格。您可以将它附加到一个 GameObject 上,然后通过检查器中的参数进行自定义设置。
相关推荐
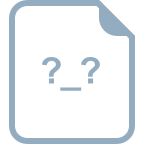
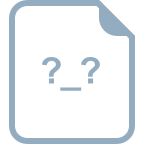














