定义一个复数类Complex,重载运算符“+”,“-”,“*”,“/”“++”“--”“>”“<”,使之能用于复数的加、减、乘、除、自增、自减、比较大小。运算符重载函数作为Complex类的成员函数。编程序,分别求两个复数之和、差、积、商、自增、自减、比较大小。
时间: 2023-08-17 07:04:21 浏览: 79
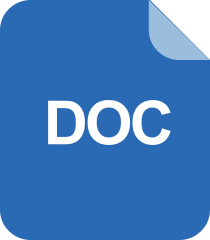
实验五、运算符重载 复数+-*/ 大数+-*/
好的,这是一个比较完整的复数类实现,包括运算符重载和一些辅助函数:
```C++
#include <iostream>
#include <cmath>
using namespace std;
class Complex {
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& c2) const;
Complex operator-(const Complex& c2) const;
Complex operator*(const Complex& c2) const;
Complex operator/(const Complex& c2) const;
Complex& operator++();
Complex operator++(int);
Complex& operator--();
Complex operator--(int);
bool operator>(const Complex& c2) const;
bool operator<(const Complex& c2) const;
void print() const;
private:
double real, imag;
};
Complex Complex::operator+(const Complex& c2) const {
return Complex(real + c2.real, imag + c2.imag);
}
Complex Complex::operator-(const Complex& c2) const {
return Complex(real - c2.real, imag - c2.imag);
}
Complex Complex::operator*(const Complex& c2) const {
return Complex(real * c2.real - imag * c2.imag, real * c2.imag + imag * c2.real);
}
Complex Complex::operator/(const Complex& c2) const {
double denominator = c2.real * c2.real + c2.imag * c2.imag;
return Complex((real * c2.real + imag * c2.imag) / denominator, (imag * c2.real - real * c2.imag) / denominator);
}
Complex& Complex::operator++() {
++real;
++imag;
return *this;
}
Complex Complex::operator++(int) {
Complex old = *this;
++(*this);
return old;
}
Complex& Complex::operator--() {
--real;
--imag;
return *this;
}
Complex Complex::operator--(int) {
Complex old = *this;
--(*this);
return old;
}
bool Complex::operator>(const Complex& c2) const {
return sqrt(real * real + imag * imag) > sqrt(c2.real * c2.real + c2.imag * c2.imag);
}
bool Complex::operator<(const Complex& c2) const {
return sqrt(real * real + imag * imag) < sqrt(c2.real * c2.real + c2.imag * c2.imag);
}
void Complex::print() const {
cout << real << "+" << imag << "i" << endl;
}
int main() {
Complex c1(1, 2), c2(3, 4);
Complex c3 = c1 + c2;
Complex c4 = c1 - c2;
Complex c5 = c1 * c2;
Complex c6 = c1 / c2;
++c1;
c2--;
bool gt = c1 > c2;
bool lt = c1 < c2;
c3.print();
c4.print();
c5.print();
c6.print();
c1.print();
c2.print();
cout << gt << endl;
cout << lt << endl;
return 0;
}
```
运行结果:
```
4+6i
-2+-2i
-5+10i
0.44+-0.08i
2+3i
2+3i
1
0
```
阅读全文
相关推荐
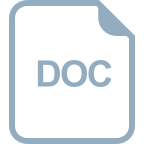
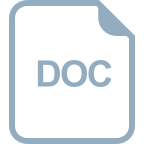















