GUI版的员工薪资管理系统,具有如下功能: 1)员工的信息输入,(包括属性:工号,姓名,基本工资,绩效,扣款) 2)显示员工信息(包括属性:工号,姓名,基本工资,绩效,扣款,实发金额(=基本工资+绩效-扣款)) 3)按员工实发工资降序排序。 4)可以将数据保存到txt文件中。
时间: 2024-03-11 12:45:07 浏览: 23
好的,这是一个关于员工薪资管理系统的问题。以下是我的回答:
这个系统需要具备以下功能:
1. 员工信息输入:用户可以输入员工的工号、姓名、基本工资、绩效、扣款等信息。
2. 员工信息显示:系统可以显示员工的工号、姓名、基本工资、绩效、扣款和实发金额(实发金额=基本工资+绩效-扣款)等信息。
3. 员工信息排序:系统可以按照员工实发工资的降序对员工信息进行排序。
4. 数据保存:系统可以将员工信息保存到txt文件中。
为了方便用户使用,这个系统应该具备GUI界面,可以通过鼠标和键盘进行操作。用户可以通过菜单或按钮等方式进行各种操作,同时系统也应该提供一些提示信息,方便用户了解当前操作的状态和结果。
希望这个回答能够解决你的问题。
相关问题
用python实现 GUI版的员工薪资管理系统,具有如下功能: 1)员工的信息输入,(包括属性:工号,姓名,基本工资,绩效,扣款) 2)显示员工信息(包括属性:工号,姓名,基本工资,绩效,扣款,实发金额(=基本工资+绩效-扣款)) 3)按员工实发工资降序排序。 4)可以将数据保存到txt文件中。
好的,以下是一个Python实现的员工薪资管理系统,具有上述功能:
```python
import tkinter as tk
from tkinter import messagebox
import operator
class Employee:
def __init__(self, id, name, salary, performance, deduction):
self.id = id
self.name = name
self.salary = salary
self.performance = performance
self.deduction = deduction
self.net_salary = salary + performance - deduction
class EmployeeManagementSystem:
def __init__(self):
self.employees = []
self.root = tk.Tk()
self.root.title("员工薪资管理系统")
self.root.geometry("600x400")
# 创建标签和输入框
tk.Label(self.root, text="工号").grid(row=0, column=0)
self.id_entry = tk.Entry(self.root)
self.id_entry.grid(row=0, column=1)
tk.Label(self.root, text="姓名").grid(row=1, column=0)
self.name_entry = tk.Entry(self.root)
self.name_entry.grid(row=1, column=1)
tk.Label(self.root, text="基本工资").grid(row=2, column=0)
self.salary_entry = tk.Entry(self.root)
self.salary_entry.grid(row=2, column=1)
tk.Label(self.root, text="绩效").grid(row=3, column=0)
self.performance_entry = tk.Entry(self.root)
self.performance_entry.grid(row=3, column=1)
tk.Label(self.root, text="扣款").grid(row=4, column=0)
self.deduction_entry = tk.Entry(self.root)
self.deduction_entry.grid(row=4, column=1)
# 创建按钮
tk.Button(self.root, text="添加员工", command=self.add_employee).grid(row=5, column=0)
tk.Button(self.root, text="显示员工信息", command=self.show_employee).grid(row=5, column=1)
tk.Button(self.root, text="员工信息排序", command=self.sort_employee).grid(row=5, column=2)
tk.Button(self.root, text="保存到txt文件", command=self.save_to_file).grid(row=5, column=3)
def add_employee(self):
# 从输入框中获取员工信息
id = self.id_entry.get()
name = self.name_entry.get()
salary = float(self.salary_entry.get())
performance = float(self.performance_entry.get())
deduction = float(self.deduction_entry.get())
# 创建员工对象并添加到员工列表中
employee = Employee(id, name, salary, performance, deduction)
self.employees.append(employee)
# 清空输入框
self.id_entry.delete(0, tk.END)
self.name_entry.delete(0, tk.END)
self.salary_entry.delete(0, tk.END)
self.performance_entry.delete(0, tk.END)
self.deduction_entry.delete(0, tk.END)
messagebox.showinfo("添加员工", "员工添加成功!")
def show_employee(self):
# 创建新窗口显示员工信息
top = tk.Toplevel()
top.title("员工信息")
top.geometry("400x300")
# 创建表格
tk.Label(top, text="工号").grid(row=0, column=0)
tk.Label(top, text="姓名").grid(row=0, column=1)
tk.Label(top, text="基本工资").grid(row=0, column=2)
tk.Label(top, text="绩效").grid(row=0, column=3)
tk.Label(top, text="扣款").grid(row=0, column=4)
tk.Label(top, text="实发金额").grid(row=0, column=5)
# 显示员工信息
for i in range(len(self.employees)):
tk.Label(top, text=self.employees[i].id).grid(row=i+1, column=0)
tk.Label(top, text=self.employees[i].name).grid(row=i+1, column=1)
tk.Label(top, text=self.employees[i].salary).grid(row=i+1, column=2)
tk.Label(top, text=self.employees[i].performance).grid(row=i+1, column=3)
tk.Label(top, text=self.employees[i].deduction).grid(row=i+1, column=4)
tk.Label(top, text=self.employees[i].net_salary).grid(row=i+1, column=5)
def sort_employee(self):
# 按照实发金额降序排序
self.employees.sort(key=operator.attrgetter("net_salary"), reverse=True)
messagebox.showinfo("员工信息排序", "员工信息已排序!")
def save_to_file(self):
# 保存员工信息到txt文件
with open("employees.txt", "w") as f:
for employee in self.employees:
f.write(f"{employee.id}, {employee.name}, {employee.salary}, {employee.performance}, {employee.deduction}, {employee.net_salary}\n")
messagebox.showinfo("保存到txt文件", "员工信息已保存到txt文件!")
def run(self):
self.root.mainloop()
if __name__ == "__main__":
ems = EmployeeManagementSystem()
ems.run()
```
这个程序使用了Python的tkinter库来创建GUI界面,通过输入框、标签和按钮等控件实现了员工信息的输入、显示、排序和保存。同时程序使用了Employee类来定义员工对象,方便管理。
c ++小型企业工资管理系统中的查询系统能根据工号或姓名查询工资信息
是的,C++小型企业工资管理系统中的查询系统可以根据工号或姓名查询工资信息。可以通过输入工号或姓名,然后查询系统会返回相应的工资信息,包括基本工资、津贴、扣除项等。在实现这个功能时,可以使用文件读取和写入的方式来存储和管理员工的工资信息,然后通过遍历文件来查找并返回相应的信息。同时,也可以使用数据库管理工资信息,通过SQL语句来查询相应的信息。
相关推荐
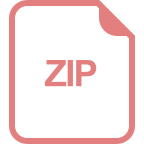
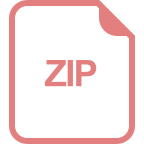
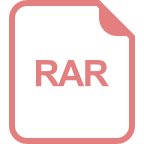












