torch.bmm(a, b)对应的keras代码
时间: 2024-03-05 11:54:38 浏览: 18
`torch.bmm(a, b)`计算两个3D张量的批量矩阵乘法。如果`a`的形状为`(batch_size, n, p)`,`b`的形状为`(batch_size, p, m)`,则输出的形状为`(batch_size, n, m)`。对应的keras代码如下:
```python
import tensorflow as tf
# 假设a和b是形状为(batch_size, n, p)和(batch_size, p, m)的张量
a = tf.random.normal((3, 4, 5))
b = tf.random.normal((3, 5, 6))
# 计算两个张量的批量矩阵乘法
c = tf.matmul(a, b)
# 打印输出张量的形状
print(c.shape)
```
其中,`tf.matmul`函数用于计算两个张量的矩阵乘法,第一个参数是左边的张量`a`,第二个参数是右边的张量`b`。在这里,`a`和`b`的形状分别是`(3, 4, 5)`和`(3, 5, 6)`,因此输出的张量`c`的形状是`(3, 4, 6)`,其中`batch_size=3`,`n=4`,`p=5`,`m=6`。打印输出张量的形状可以使用`c.shape`。
相关问题
torch.bmm(a, b)
torch.bmm(a, b)的作用是进行批量的矩阵乘法操作。其中a的维度是b * m * n,b的维度是b * n * p,结果的维度是b * m * p。这意味着对于每一个批次中的矩阵a和b,会进行矩阵乘法操作,输出一个结果矩阵。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [【torch小知识点03】矩阵乘法总结](https://blog.csdn.net/wistonty11/article/details/128758903)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
torch.dot和torch.bmm的区别
torch.dot是PyTorch中的一个函数,用于计算两个张量的点积。点积是将两个向量的对应元素相乘,并将结果相加得到的标量值。它适用于一维张量。
而torch.bmm是PyTorch中的一个函数,用于计算两个批次的矩阵乘法。它接受两个三维张量作为输入,其中第一个张量的形状为(batch_size, n, m),第二个张量的形状为(batch_size, m, p),返回的结果是一个形状为(batch_size, n, p)的张量。bmm代表的是batch matrix multiplication,可以同时对多个矩阵进行乘法运算。
总结一下:
- torch.dot适用于一维张量,计算两个向量的点积,返回一个标量值。
- torch.bmm适用于三维张量,计算两个批次的矩阵乘法,返回一个三维张量。
相关推荐
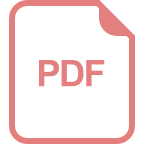
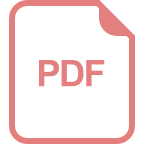












