rsa加解密全过程代码
时间: 2024-09-04 13:00:39 浏览: 45
RSA加密和解密的过程涉及到很多细节,包括密钥生成、加载以及实际的加密/解密操作。这里提供了一个简单的示例,使用OpenSSL库来演示RSA加密和解密过程:
```c
#include <stdio.h>
#include <string.h>
#include <openssl/evp.h>
#include <openssl/pem.h>
#include <openssl/err.h>
// 加载公钥和私钥
void load_keys(const char *public_pem_path, const char *private_pem_path) {
BIO *bio_pub, *bio_priv;
RSA *rsa_pub, *rsa_priv;
bio_pub = BIO_new_file(public_pem_path, "r");
bio_priv = BIO_new_file(private_pem_path, "r");
rsa_pub = PEM_read_bio_RSAPublicKey(bio_pub, NULL, NULL, NULL);
rsa_priv = PEM_read_bio_RSAPrivateKey(bio_priv, NULL, NULL, NULL);
if (!rsa_pub || !rsa_priv) {
perror("Error loading keys");
exit(1);
}
// 程序里可以在这里保存密钥,如果需要长期使用
// 这里只是为了演示,不再保存
BIO_free_all(bio_pub);
BIO_free_all(bio_priv);
}
// 加密函数
char *encrypt_rsa(RSA *rsa_pub, const char *plaintext) {
BIO *bio_out;
unsigned char encrypted_data[EVP_MAX_MD_SIZE + 128];
int encrypted_len;
bio_out = BIO_new(BIO_s_mem());
if (!BIO_write(bio_out, plaintext, strlen(plaintext))) {
BIO_free_all(bio_out);
return NULL;
}
if (RSA_public_encrypt(strlen(plaintext), bio_out->data, encrypted_data, rsa_pub, RSA_PKCS1_OAEP_PADDING) <= 0) {
BIO_free_all(bio_out);
return NULL;
}
encrypted_len = bio_out->length;
encrypted_data[encrypted_len] = '\0'; // 添加终止符
BIO_free_all(bio_out);
return (char *) encrypted_data;
}
// 解密函数
char *decrypt_rsa(RSA *rsa_priv, const char *ciphertext) {
BIO *bio_in = BIO_new_mem_buf((uint8_t *) ciphertext, strlen(ciphertext));
unsigned char decrypted_data[EVP_MAX_MD_SIZE + 128];
int decrypted_len;
if (RSA_private_decrypt(strlen(ciphertext), bio_in->data, decrypted_data, rsa_priv, RSA_PKCS1_OAEP_PADDING) <= 0) {
BIO_free_all(bio_in);
return NULL;
}
decrypted_len = RSA_size(rsa_priv);
decrypted_data[decrypted_len] = '\0'; // 添加终止符
BIO_free_all(bio_in);
return (char *) decrypted_data;
}
int main() {
load_keys("path_to_public.pem", "path_to_private.pem"); // 替换为实际路径
char *plaintext = "Hello, RSA!";
char *encrypted = encrypt_rsa(<RSA_PUB_KEY>, plaintext);
char *decrypted = decrypt_rsa(<RSA_PRIV_KEY>, encrypted); // 用实际的私钥替换
if (decrypted) {
printf("Encrypted: %s\n", encrypted);
printf("Decrypted: %s\n", decrypted);
} else {
printf("Failed to encrypt or decrypt.\n");
}
free(encrypted);
free(decrypted);
return 0;
}
```
注意:这个例子假设了公钥和私钥文件已存在,并且`<RSA_PUB_KEY>`和`<RSA_PRIV_KEY>`需要替换为你实际的RSA密钥变量。在实际项目中,你需要处理可能出现的各种错误情况。
阅读全文
相关推荐
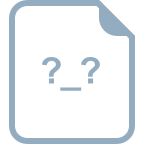
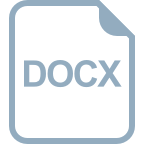
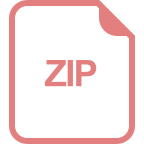















