输出队列中最后一个元素
时间: 2024-06-13 17:05:28 浏览: 97
根据提供的引用内容,无法直接回答输出队列中最后一个元素的问题。但是,可以根据提供的引用内容给出一个输出队列中所有元素的代码示例,供您参考:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 10
struct Queue {
int Data[MAX_QUEUE_SIZE];
int Front;
int Rear;
};
void InitQueue(struct Queue* Q) {
Q->Front = Q->Rear = 0;
}
int IsFull(struct Queue* Q) {
return ((Q->Rear + 1) % MAX_QUEUE_SIZE == Q->Front);
}
int IsEmpty(struct Queue* Q) {
return (Q->Front == Q->Rear);
}
void EnQueue(struct Queue* Q, int X) {
if (IsFull(Q)) {
printf("Queue is full\n");
return;
}
Q->Rear = (Q->Rear + 1) % MAX_QUEUE_SIZE;
Q->Data[Q->Rear] = X;
}
int DeQueue(struct Queue* Q) {
if (IsEmpty(Q)) {
printf("Queue is empty\n");
return -1;
}
Q->Front = (Q->Front + 1) % MAX_QUEUE_SIZE; return Q->Data[Q->Front];
}
void Print(struct Queue* Q) {
int k = Q->Front;
while (k != Q->Rear) {
printf("%d ", Q->Data[k]);
k++;
}
printf("%d\n", Q->Data[k]);
}
int main() {
struct Queue Q[MAX_QUEUE_SIZE];
int n, i, j, x;
for (i = 0; i < MAX_QUEUE_SIZE; i++) {
InitQueue(&Q[i]);
}
printf("请输入n个10以内的数:\n");
scanf("%d", &n);
for (i = 0; i < n; i++) {
scanf("%d", &x);
j = x % MAX_QUEUE_SIZE;
EnQueue(&Q[j], x);
}
printf("非空队列中的元素为:\n");
for (i = 0; i < MAX_QUEUE_SIZE; i++) {
if (!IsEmpty(&Q[i])) {
Print(&Q[i]);
}
}
return 0;
}
```
该代码示例可以实现输入n个10以内的数,将它们插入到对应的队列中,并输出非空队列中的所有元素。如果您需要输出队列中最后一个元素,可以在Print函数中进行修改,使其只输出队列中最后一个元素即可。
阅读全文
相关推荐
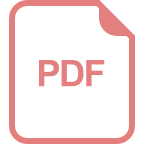
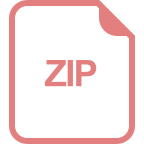
















