数据结构(C语言)算法设计:假设以带头结点的循环链表表示队列,并且只设一个指向尾结点的指针,不设头指针,写出队列初始化、判断队列是否为空、入队和出队等算法。
时间: 2024-11-27 16:22:56 浏览: 38
在C语言中,使用单链表作为循环队列的数据结构可以这样设计:
1. **队列初始化**:
- 初始化一个空的循环链表。创建一个新的节点作为尾部,并将其next指向自身,形成一个环。
```c
struct Node {
int data;
struct Node *next;
};
void initializeQueue(struct Node **rear) {
*rear = NULL; // 初始化尾指针为NULL
}
```
2. **判断队列是否为空**:
- 判断队列为空就是看尾指针是否为NULL。
```c
bool isEmpty(struct Node *rear) {
return rear == NULL;
}
```
3. **入队操作(enqueue)**:
- 新节点插入到当前尾节点之后。
```c
void enqueue(struct Node **rear, int data) {
if (*rear != NULL) {
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = *rear;
*rear = newNode;
} else {
// 队列初始时直接添加新节点并设置尾部
*rear = newNode;
}
}
```
4. **出队操作(dequeue)**:
- 如果队列非空,移除并返回头部元素,然后更新尾指针到下一个节点。
```c
int dequeue(struct Node **front, struct Node **rear) {
if (isEmpty(*front)) {
return -1; // 返回错误代码,队列为空
}
int data = (*front)->data;
struct Node *temp = *front;
*front = (*front)->next;
if (*front == *rear) { // 清空队列后更新尾部指针
*rear = NULL;
}
free(temp); // 释放已处理的节点内存
return data;
}
```
阅读全文
相关推荐
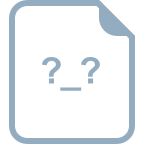
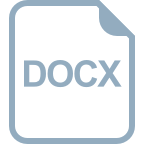
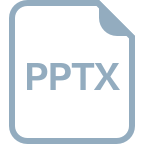















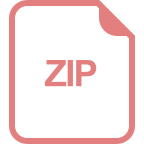
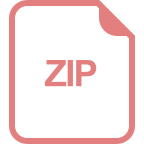