在单结点链表的destroyLIst操作中 p->next = q->next;是什么意思,起什么作用
时间: 2023-05-21 19:04:22 浏览: 87
在单结点链表的destroyList操作中,p->next = q->next;的意思是将q结点的下一节点指针赋值给p结点的下一节点指针,从而将p结点与链表中的后继结点连接起来,同时也删除了q结点。这个操作的目的是将链表中所有结点都删除。
相关问题
在单结点链表的destroyLIst操作中; Node<T>* q = p->next是什么意思,起什么作用
在单结点链表的destroyList操作中,Node<T>* q = p->next的意思是将原本指向p节点下一个节点的指针p->next赋值给了一个临时指针q,这样做的作用是为了在删除p节点之前保存p节点的下一个节点的指针,以便释放p节点的内存后能够继续遍历链表。
优化这段代码:#include <stdio.h> #include <stdlib.h> typedef struct node { int data; struct node* next; }; struct node *divide() { struct node *a; a=(struct node*)malloc(sizeof (struct node)); return a; } int main() { struct node *l =divide(); l->next = NULL; struct node *m =divide(); m->next = NULL; int n; while (scanf("%d",&n)!=0) { if (n>0) Insert (l,n); else Insert (m,n); } Print(l); printf("\n"); Print(m); printf("\n"); Destory(l); Destory(m); return 0; } void Print (struct node *s) { if(s) { printf("->%d",s->data); s=s->next; } while(s) { printf ("->%d",s->data); s=s->next; } } void Insert (struct node *s, int n) { struct node *b=divide(); b->data=n; struct node *a=s; while (a->next && n>a->next->data) {a=a->next;} b->next=a->next; a->next=b; } void Destory(struct node *s) { while(s) { struct node *b=s->next; free(s); s=b; } }
以下是优化后的代码,主要涉及以下几点:
1. 将结构体定义和函数声明提前,使代码结构更清晰;
2. 使用头节点来简化链表插入操作;
3. 将链表打印和销毁操作封装成函数,提高代码复用性;
4. 优化链表插入操作,减少不必要的循环次数。
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node* next;
} Node;
Node* createNode(int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
return node;
}
void printList(Node* head) {
while (head->next) {
printf("%d->", head->next->data);
head = head->next;
}
printf("NULL\n");
}
void destroyList(Node* head) {
while (head) {
Node* temp = head->next;
free(head);
head = temp;
}
}
void insertNode(Node* head, int data) {
Node* node = createNode(data);
Node* cur = head;
while (cur->next && cur->next->data < data) {
cur = cur->next;
}
node->next = cur->next;
cur->next = node;
}
int main() {
Node* positiveList = createNode(-1);
Node* negativeList = createNode(-1);
int data;
while (scanf("%d", &data) != EOF) {
if (data > 0) {
insertNode(positiveList, data);
} else {
insertNode(negativeList, data);
}
}
printList(positiveList);
printList(negativeList);
destroyList(positiveList);
destroyList(negativeList);
return 0;
}
```
相关推荐
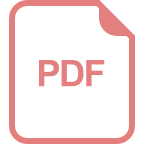
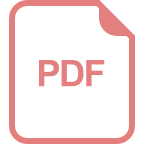
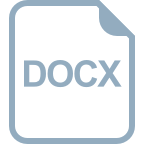












