编写函数,通过键盘接收正整数n,计算1-2+3-4
时间: 2024-06-06 07:11:18 浏览: 125
def calc(n):
res = 0
for i in range(1, n+1, 2):
res += i
for j in range(2, n+1, 2):
res -= j
return res
n = int(input("请输入正整数n:"))
print("1-2+3-4+...+n的结果为:", calc(n))
相关问题
运用for循环,sum求和函数,reduce函数,while 循环,编写程序,接收用户从键盘输入的正整数n,输出1+2+.-+n 的和。
当然可以。在Python中,你可以使用多种方法来实现这个需求,以下是三种不同的解决方案:
1. **Using for loop and sum function**:
```python
def calculate_sum(n):
return sum(range(1, n + 1))
n = int(input("请输入一个正整数:"))
print("1到{}的和是:{}".format(n, calculate_sum(n)))
```
2. **Using reduce function from functools module**:
```python
from functools import reduce
def add(x, y):
return x + y
n = int(input("请输入一个正整数:"))
total = reduce(add, range(1, n + 1))
print("1到{}的和是:{}".format(n, total))
```
3. **Using while loop**:
```python
def calculate_sum_loop(n):
total = 0
i = 1
while i <= n:
total += i
i += 1
return total
n = int(input("请输入一个正整数:"))
print("1到{}的和是:{}".format(n, calculate_sum_loop(n)))
```
在这三个例子中,用户都可以通过键盘输入正整数`n`,然后程序会计算并打印出从1加到n的所有数字的和。
设计一个子程序,计算1+2++N,其中N为给定的正整数,且N小于100。 2. 定义10个字型带符号数,编写子程序分别求其最大值和统计正数的个数。 3. 从键盘接收4位十进制数,将其转换为十六进制数并显示。汇编语言
设计一个子程序(在汇编语言中通常称为子例行程或函数)来完成这三个任务:
1. 计算1到N的和(例如,1 + 2 + ... + N):
```assembly
; 使用x86汇编语言(如Intel x86)
section .data
N db 99 ; 假设N的最大值为99
result dw 0 ; 结果存储在这里
calculate_sum proc near
push bp ; 设置基址寄存器
mov bp, sp ; 保存堆栈指针
xor ax, ax ; 初始化累加器
mov cx, [bp+N] ; 读取N
loop_start:
add ax, cx ; 累加
loop loop_start ; 减少cx,直到为0,继续循环
pop bp ; 恢复基址寄存器
ret ; 返回结果到调用者处
calculate_sum endp
```
2. 寻找字节变量中的最大值和统计正数个数:
```assembly
find_max_and_count proc near
; 定义数组和变量
section .bss
numbers[10] db -1, -2, ... , -10 (填充负数示例)
max_result db ?
positive_count db 0
; 遍历数组,找到最大值和计数正数
mov di, offset numbers ; 使用DI作为偏移地址
mov al, [di] ; 用AL暂存第一个元素
inc di ; 移动到下一个元素
max_loop:
cmp al, [di] ; 如果当前元素大于之前的最大值
jle not_greater
mov max_result, al ; 更新最大值
not_greater:
cmp [di], 0 ; 判断是否为正数
jg increment_positive
jmp next_element
increment_positive:
inc positive_count ; 增加正数计数
next_element:
inc di ; 移动到下一个元素
cmp di, 10 ; 到达数组结束则退出循环
jne max_loop
; 返回最大值和正数个数
mov ax, max_result
mov bx, positive_count
pop bp
ret
find_max_and_count endp
```
3. 将4位十进制数转为十六进制并显示:
```assembly
convert_to_hex proc near
; 假设输入在eax寄存器中
; ... (实际的转换过程略复杂,需要使用除法和模运算)
; 保存结果在ebx寄存器中,假设它是1A (16进制)
; 显示十六进制数
; 这里需要一个输出字符串缓冲区和显示函数的具体实现(取决于具体环境),这里省略
; 一般会使用INT 21H系统调用来打印字符
pop bp
ret
convert_to_hex endp
```
阅读全文
相关推荐
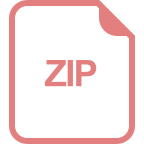
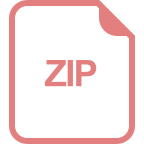
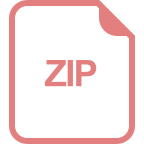
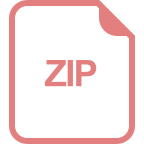
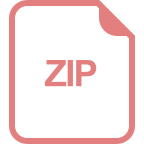









