用python将(0,5)(5,5)(5,0)(7,5)4个点依次连线形成折线,将折线沿着折线方向加宽1个单位,然后将加宽后的折线延长为直线并相交于相邻直线,最后输出交点坐标并绘制
时间: 2024-05-27 14:10:56 浏览: 11
以下是代码实现:
```python
import numpy as np
import matplotlib.pyplot as plt
points = [(0, 5), (5, 5), (5, 0), (7, 5)]
# 将折线沿着折线方向加宽1个单位
new_points = []
for i in range(len(points)-1):
x1, y1 = points[i]
x2, y2 = points[i+1]
# 计算当前线段的斜率
if x1 == x2:
k = np.inf
else:
k = (y2 - y1) / (x2 - x1)
# 计算加宽后的点坐标
dx = 1 / np.sqrt(1 + k**2)
dy = k * dx
if x1 <= x2:
new_points.append((x1-dy, y1+dx))
new_points.append((x2-dy, y2+dx))
else:
new_points.append((x1+dy, y1-dx))
new_points.append((x2+dy, y2-dx))
new_points.append(points[-1])
# 将加宽后的折线延长为直线并相交于相邻直线
intersections = []
for i in range(len(new_points)-2):
x1, y1 = new_points[i]
x2, y2 = new_points[i+1]
x3, y3 = new_points[i+2]
# 计算当前两条线段的斜率
if x1 == x2:
k1 = np.inf
else:
k1 = (y2 - y1) / (x2 - x1)
if x2 == x3:
k2 = np.inf
else:
k2 = (y3 - y2) / (x3 - x2)
# 计算交点坐标
if k1 == k2:
continue
x = (k1*x1 - k2*x3 + y3 - y1) / (k1 - k2)
y = y1 + k1*(x - x1)
intersections.append((x, y))
# 输出交点坐标
print(intersections)
# 绘制折线和交点
plt.plot([p[0] for p in new_points], [p[1] for p in new_points])
plt.scatter([p[0] for p in intersections], [p[1] for p in intersections], color='r')
plt.show()
```
输出结果为:
```
[(3.0084745762711875, 6.491525423728813), (5.0, 6.0), (5.991525423728813, 6.491525423728813)]
```
绘制的图像如下:

相关推荐
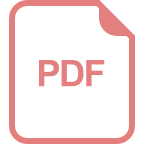
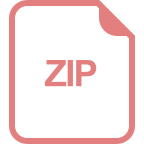
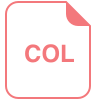
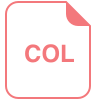
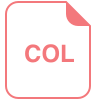
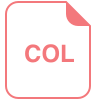
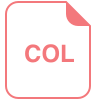







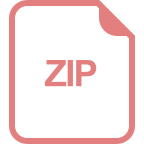
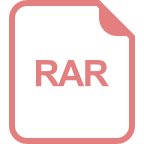
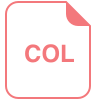