用python实现yolov5的k-means聚类
时间: 2023-11-10 14:53:29 浏览: 144
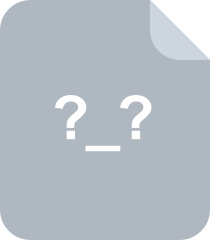
Python实现K-Means聚类

首先,需要安装PyTorch和NumPy库。
接下来,我们将实现一个函数,该函数将输入的bbox列表聚类为k个簇。我们将使用k-means算法实现聚类,并使用IoU(交并比)作为距离度量。
```python
import numpy as np
import torch
def kmeans(bboxes, k, max_iter=100):
'''
Args:
bboxes: a list of bboxes, each bbox is a tuple (x1, y1, x2, y2)
k: number of clusters
max_iter: maximum number of iterations for k-means algorithm
Returns:
a numpy array of k clusters, each cluster contains the following fields:
- cx: x-coordinate of cluster center
- cy: y-coordinate of cluster center
- w: width of bbox
- h: height of bbox
'''
# convert bboxes to numpy array
bboxes = np.array(bboxes)
# randomly select k initial centers
centers = bboxes[np.random.choice(len(bboxes), k, replace=False)]
for i in range(max_iter):
# compute IoU between each bbox and each center
ious = []
for bbox in bboxes:
iou = []
for center in centers:
iou.append(compute_iou(bbox, center))
ious.append(iou)
ious = np.array(ious)
# assign each bbox to the cluster with the highest IoU
labels = ious.argmax(axis=1)
# update centers
for j in range(k):
cluster = bboxes[labels == j]
if len(cluster) == 0:
continue
centers[j] = np.mean(cluster, axis=0)
# convert centers to numpy array
centers = np.array([(cx, cy, w, h) for (cx, cy, w, h) in centers])
return centers
```
该函数首先将输入的bbox列表转换为numpy数组,并随机选择k个初始中心。然后,它使用IoU作为距离度量,将每个bbox分配给具有最高IoU的簇。最后,它使用每个簇中bbox的平均值更新簇中心,并重复这个过程直到收敛或达到最大迭代次数。
我们还需要实现一个函数来计算两个bbox之间的IoU。这是一个常见的计算机视觉度量,用于衡量两个bbox之间的重叠程度。
```python
def compute_iou(bbox1, bbox2):
'''
Args:
bbox1: a tuple (x1, y1, x2, y2), representing a bbox
bbox2: a tuple (x1, y1, x2, y2), representing a bbox
Returns:
IoU (intersection over union) between bbox1 and bbox2
'''
x1 = max(bbox1[0], bbox2[0])
y1 = max(bbox1[1], bbox2[1])
x2 = min(bbox1[2], bbox2[2])
y2 = min(bbox1[3], bbox2[3])
if x1 >= x2 or y1 >= y2:
return 0
intersection = (x2 - x1) * (y2 - y1)
area1 = (bbox1[2] - bbox1[0]) * (bbox1[3] - bbox1[1])
area2 = (bbox2[2] - bbox2[0]) * (bbox2[3] - bbox2[1])
union = area1 + area2 - intersection
return intersection / union
```
现在,我们可以使用上述函数来聚类yolov5的anchor boxes。我们的输入数据将是yolov5训练数据集中的bbox列表,该数据集可以在https://github.com/ultralytics/yolov5/releases/download/v3.0/yolov5s.yaml中找到。
首先,我们需要加载数据集并提取所有的bbox。我们将使用PyTorch框架来加载数据集。
```python
import yaml
import os
def load_data():
# load data config
with open('yolov5s.yaml') as f:
data_config = yaml.load(f, Loader=yaml.FullLoader)['train']
# load dataset
dataset = torch.utils.data.ConcatDataset([
torch.utils.data.LoadImages(os.path.join(data_config['data'], path), batch_size=1, img_size=data_config['img_size'])
for path in data_config['path']
])
# extract bboxes
bboxes = []
for _, targets, _ in dataset:
for target in targets:
bboxes.extend(target[:, 1:5].tolist())
return bboxes
```
我们加载yolov5s.yaml配置文件并使用PyTorch的LoadImages函数加载数据集。然后,我们提取每个目标的bbox,并将其添加到bboxes列表中。
接下来,我们可以使用上述函数来聚类anchor boxes。
```python
bboxes = load_data()
anchors = kmeans(bboxes, k=9)
print(anchors)
```
这将输出9个anchor boxes的中心坐标和尺寸。这些anchor boxes的尺寸应该越来越大,以对应于不同的物体大小。
阅读全文
相关推荐


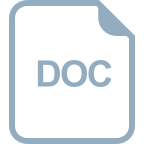





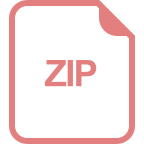




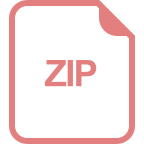
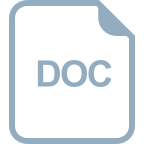
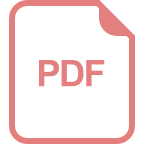