#define READ_DRV1() gpio_input_bit_get(DRIVER1_R_GPIO_Port, DRIVER1_R_Pin) #define READ_DRV2() gpio_input_bit_get(DRIVER2_R_GPIO_Port, DRIVER2_R_Pin) #define READ_DRV3() gpio_input_bit_get(DRIVER3_R_GPIO_Port, DRIVER3_R_Pin) #define READ_DRV4() gpio_input_bit_get(DRIVER4_R_GPIO_Port, DRIVER4_R_Pin) #define READ_DRV5() gpio_input_bit_get(DRIVER5_R_GPIO_Port, DRIVER5_R_Pin) #define READ_DRV6() gpio_input_bit_get(DRIVER6_R_GPIO_Port, DRIVER6_R_Pin) #define READ_DRV7() gpio_input_bit_get(DRIVER7_R_GPIO_Port, DRIVER7_R_Pin) #define READ_DRV8() gpio_input_bit_get(DRIVER8_R_GPIO_Port, DRIVER8_R_Pin) #define READ_DRV9() gpio_input_bit_get(DRIVER9_R_GPIO_Port, DRIVER9_R_Pin) #define READ_DRV10() gpio_input_bit_get(DRIVER10_R_GPIO_Port, DRIVER10_R_Pin) #define READ_DRV11() gpio_input_bit_get(DRIVER11_R_GPIO_Port, DRIVER11_R_Pin) #define READ_DRV12() gpio_input_bit_get(DRIVER12_R_GPIO_Port, DRIVER12_R_Pin) READ_DRV如何使用for循环
时间: 2023-07-29 10:13:40 浏览: 141
可以使用for循环和宏定义来简化代码,如下所示:
```
#define READ_DRV(i) gpio_input_bit_get(DRIVER##i##_R_GPIO_Port, DRIVER##i##_R_Pin)
for (int i = 1; i <= 12; i++) {
int drv = READ_DRV(i);
// 处理drv的值
}
```
在这个例子中,我们定义了一个宏定义`READ_DRV(i)`,用于根据输入的参数i读取对应的GPIO引脚状态。在for循环中,我们循环遍历12个引脚,依次读取它们的状态并进行处理。
需要注意的是,宏定义中使用了双井号(##)运算符,用于将参数i与固定的字符串DRIVER进行拼接,形成对应的GPIO引脚的名称。这样,我们就可以使用一个宏定义来简化对多个GPIO引脚的读取操作。
相关问题
#include <rtthread.h> #include <drv_lcd.h> #include <board.h> #include <rthw.h> #include <rtdevice.h> #include <drv_gpio.h> #include <rtdbg.h> 请用以上头文件完成我的要求,我的要求是请使用RT-Thread实时操作系统编写的C语言程序,并利用了几个驱动程序,如DRV_LCD和DRV_GPIO,来控制LCD屏幕和GPIO引脚,实现一个俄罗斯方块的游戏,程序用多线程处理不同任务,此外使用邮箱在线程之间进行通信,并使用定时器以固定间隔更新游戏状态。最后控制RGB LED和蜂鸣器以向用户提供视觉和听觉反馈。
这是一个比较复杂的项目,需要一定的嵌入式开发经验。以下是一个简单的示例代码,仅供参考:
```c
#include <rtthread.h>
#include <drv_lcd.h>
#include <board.h>
#include <rthw.h>
#include <rtdevice.h>
#include <drv_gpio.h>
#include <rtdbg.h>
#define LCD_WIDTH 240
#define LCD_HEIGHT 320
#define BLOCK_SIZE 20
static rt_uint8_t block_colors[7][3] = {
{255, 0, 0}, // 红色
{255, 165, 0}, // 橙色
{255, 255, 0}, // 黄色
{0, 128, 0}, // 绿色
{0, 0, 255}, // 蓝色
{75, 0, 130}, // 紫色
{128, 0, 128} // 粉色
};
static rt_uint8_t game_board[LCD_WIDTH / BLOCK_SIZE][LCD_HEIGHT / BLOCK_SIZE];
static rt_uint8_t cur_block[4][4];
static rt_uint8_t cur_block_color[3];
static rt_uint8_t cur_block_x, cur_block_y;
static rt_uint8_t cur_block_rotate;
static rt_uint8_t score;
static rt_uint8_t game_over;
static struct rt_mailbox game_mailbox;
static struct rt_semaphore lcd_sem;
static struct rt_semaphore block_sem;
static rt_device_t lcd_dev;
static rt_device_t gpio_dev;
static void lcd_clear(rt_uint8_t color)
{
rt_uint8_t *lcd_buf;
rt_uint32_t i, j;
rt_sem_take(&lcd_sem, RT_WAITING_FOREVER);
lcd_buf = rt_malloc(LCD_WIDTH * LCD_HEIGHT * 2);
for (i = 0; i < LCD_WIDTH * LCD_HEIGHT; i++)
{
lcd_buf[i * 2] = color & 0xff;
lcd_buf[i * 2 + 1] = (color >> 8) & 0xff;
}
rt_device_write(lcd_dev, 0, lcd_buf, LCD_WIDTH * LCD_HEIGHT * 2);
rt_free(lcd_buf);
rt_sem_release(&lcd_sem);
}
static void lcd_draw_block(rt_uint8_t x, rt_uint8_t y, rt_uint8_t color)
{
rt_uint8_t *lcd_buf;
rt_uint32_t i, j;
rt_sem_take(&lcd_sem, RT_WAITING_FOREVER);
lcd_buf = rt_malloc(BLOCK_SIZE * BLOCK_SIZE * 2);
for (i = 0; i < BLOCK_SIZE; i++)
{
for (j = 0; j < BLOCK_SIZE; j++)
{
if (i == 0 || i == BLOCK_SIZE - 1 || j == 0 || j == BLOCK_SIZE - 1)
{
lcd_buf[(i * BLOCK_SIZE + j) * 2] = 0xff;
lcd_buf[(i * BLOCK_SIZE + j) * 2 + 1] = 0xff;
}
else
{
lcd_buf[(i * BLOCK_SIZE + j) * 2] = color & 0xff;
lcd_buf[(i * BLOCK_SIZE + j) * 2 + 1] = (color >> 8) & 0xff;
}
}
}
rt_device_write(lcd_dev, (x + 1) * BLOCK_SIZE, (y + 1) * BLOCK_SIZE, lcd_buf, BLOCK_SIZE * BLOCK_SIZE * 2);
rt_free(lcd_buf);
rt_sem_release(&lcd_sem);
}
static void lcd_draw_board(void)
{
rt_uint8_t i, j;
for (i = 0; i < LCD_WIDTH / BLOCK_SIZE; i++)
{
for (j = 0; j < LCD_HEIGHT / BLOCK_SIZE; j++)
{
if (game_board[i][j])
{
lcd_draw_block(i, j, block_colors[game_board[i][j] - 1][0] << 16
| block_colors[game_board[i][j] - 1][1] << 8
| block_colors[game_board[i][j] - 1][2]);
}
else
{
lcd_draw_block(i, j, 0);
}
}
}
}
static rt_err_t gpio_callback(rt_device_t dev, rt_size_t size)
{
rt_uint8_t key_value;
rt_device_read(dev, 0, &key_value, 1);
switch (key_value)
{
case 0x11: // 左键
rt_sem_release(&block_sem);
break;
case 0x21: // 右键
rt_sem_release(&block_sem);
break;
case 0x41: // 上键
rt_sem_release(&block_sem);
break;
case 0x81: // 下键
rt_sem_release(&block_sem);
break;
default:
break;
}
return RT_EOK;
}
static void block_thread_entry(void *parameter)
{
rt_uint8_t i, j, k;
rt_uint8_t next_block[4][4];
rt_uint8_t next_block_color[3];
rt_uint8_t next_block_rotate;
rt_uint8_t next_block_x, next_block_y;
rt_uint8_t is_game_over;
while (1)
{
// 生成下一个方块
next_block_color[0] = block_colors[rt_tick_get() % 7][0];
next_block_color[1] = block_colors[rt_tick_get() % 7][1];
next_block_color[2] = block_colors[rt_tick_get() % 7][2];
next_block_rotate = rt_tick_get() % 4;
next_block_x = (LCD_WIDTH / BLOCK_SIZE - 4) / 2;
next_block_y = 0;
switch (rt_tick_get() % 7)
{
case 0: // I
next_block[0][0] = 0; next_block[0][1] = 0; next_block[0][2] = 0; next_block[0][3] = 0;
next_block[1][0] = 1; next_block[1][1] = 1; next_block[1][2] = 1; next_block[1][3] = 1;
next_block[2][0] = 0; next_block[2][1] = 0; next_block[2][2] = 0; next_block[2][3] = 0;
next_block[3][0] = 0; next_block[3][1] = 0; next_block[3][2] = 0; next_block[3][3] = 0;
break;
case 1: // J
next_block[0][0] = 0; next_block[0][1] = 1; next_block[0][2] = 0; next_block[0][3] = 0;
next_block[1][0] = 0; next_block[1][1] = 1; next_block[1][2] = 1; next_block[1][3] = 1;
next_block[2][0] = 0; next_block[2][1] = 0; next_block[2][2] = 0; next_block[2][3] = 0;
next_block[3][0] = 0; next_block[3][1] = 0; next_block[3][2] = 0; next_block[3][3] = 0;
break;
case 2: // L
next_block[0][0] = 0; next_block[0][1] = 0; next_block[0][2] = 0; next_block[0][3] = 1;
next_block[1][0] = 0; next_block[1][1] = 1; next_block[1][2] = 1; next_block[1][3] = 1;
next_block[2][0] = 0; next_block[2][1] = 0; next_block[2][2] = 0; next_block[2][3] = 0;
next_block[3][0] = 0; next_block[3][1] = 0; next_block[3][2] = 0; next_block[3][3] = 0;
break;
case 3: // O
next_block[0][0] = 0; next_block[0][1] = 0; next_block[0][2] = 1; next_block[0][3] = 1;
next_block[1][0] = 0; next_block[1][1] = 0; next_block[1][2] = 1; next_block[1][3] = 1;
next_block[2][0] = 0; next_block[2][1] = 0; next_block[2][2] = 0; next_block[2][3] = 0;
next_block[3][0] = 0; next_block[3][1] = 0; next_block[3][2] = 0; next_block[3][3] = 0;
break;
case 4: // S
next_block[0][0] = 0; next_block[0][1] = 0; next_block[0][2] = 1; next_block[0][3] = 1;
next_block[1][0] = 0; next_block[1][1] = 1; next_block[1][2] = 1; next_block[1][3] = 0;
next_block[2][0] = 0; next_block[2][1] = 0; next_block[2][2] = 0; next_block[2][3] = 0;
next_block[3][0] = 0; next_block[3][1] = 0; next_block[3][2] = 0; next_block[3][3] = 0;
break;
case 5: // T
next_block[0][0] = 0; next_block[0][1] = 1; next_block[0][2] = 0; next_block[0][3] = 0;
next_block[1][0] = 0; next_block[1][1] = 1; next_block[1][2] = 1; next_block[1][3] = 1;
next_block[2][0] = 0; next_block[2][1] = 0; next_block[2][2] = 0; next_block[2][3] = 0;
next_block[3][0] = 0; next_block[3][1] = 0; next_block[3][2] = 0; next_block[3][3] = 0;
break;
case 6: // Z
next_block[0][0] = 0; next_block[0][1] = 1; next_block[0][2] = 1; next_block[0][3] = 0;
next_block[1][0] = 0; next_block[1][1] = 0; next_block[1][2] = 1; next_block[1][3] = 1;
next_block[2][0] = 0; next_block[2][1] = 0; next_block[2][2] = 0; next_block[2][3] = 0;
next_block[3][0] = 0; next_block[3][1] = 0; next_block[3][2] = 0; next_block[3][3] = 0;
break;
default:
break;
}
is_game_over = 0;
// 判断游戏是否结束
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (next_block[i][j])
{
if (game_board[next_block_x + i][next_block_y + j])
{
is_game_over = 1;
break;
}
}
}
if (is_game_over)
{
break;
}
}
if (is_game_over)
{
game_over = 1;
rt_kprintf("Game Over!\n");
break;
}
// 发送消息通知LCD线程绘制下一个方块
rt_memcpy(cur_block, next_block, sizeof(cur_block));
rt_memcpy(cur_block_color, next_block_color, sizeof(cur_block_color));
cur_block_x = next_block_x;
cur_block_y = next_block_y;
cur_block_rotate = next_block_rotate;
rt_mb_send(&game_mailbox, (rt_uint32_t)1);
// 等待信号量,接收操作指令
rt_sem_take(&block_sem, RT_WAITING_FOREVER);
// 处理操作指令
switch (rt_current_thread()->event_set)
{
case 0x01: // 左移
if (cur_block_x > 0)
{
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (cur_block[i][j])
{
if (game_board[cur_block_x + i - 1][cur_block_y + j])
{
goto out;
}
}
}
}
cur_block_x--;
rt_mb_send(&game_mailbox, (rt_uint32_t)1);
}
break;
case 0x02: // 右移
if (cur_block_x < LCD_WIDTH / BLOCK_SIZE - 4)
{
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (cur_block[i][j])
{
if (game_board[cur_block_x + i + 1][cur_block_y + j])
{
goto out;
}
}
}
}
cur_block_x++;
rt_mb_send(&game_mailbox, (rt_uint32_t)1);
}
break;
case 0x04: // 旋转
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
next_block[j][3 - i] = cur_block[i][j];
}
}
for (k = 0; k < cur_block_rotate; k++)
{
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
cur_block[i][j] = next_block[i][j];
}
}
rt_memcpy(next_block, cur_block, sizeof(cur_block));
}
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (cur_block[i][j])
{
if (game_board[cur_block_x + i][cur_block_y + j])
{
goto out;
}
}
}
}
rt_memcpy(cur_block, next_block, sizeof(cur_block));
rt_mb_send(&game_mailbox, (rt_uint32_t)1);
break;
case 0x08: // 下移
while (1)
{
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (cur_block[i][j])
{
if (game_board[cur_block_x + i][cur_block_y + j + 1])
{
goto out;
}
}
}
}
cur_block_y++;
rt_mb_send(&game_mailbox, (rt_uint32_t)1);
rt_thread_delay(100);
}
break;
default:
break;
}
out:
// 将方块写入游戏区域
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (cur_block[i][j])
{
game_board[cur_block_x + i][cur_block_y + j] = cur_block[i][j];
}
}
}
}
}
static void lcd_thread_entry(void *parameter)
{
rt_uint32_t i, j, k;
rt_uint8_t lcd_buf[LCD_WIDTH * LCD_HEIGHT * 2];
//
nrf_drv_common_irq_enable
`nrf_drv_common_irq_enable`是一个来自 Nordic Semiconductor 的 nRF5 SDK 中的一个函数,主要用于在驱动中启用中断。它通常用于处理通用中断,如GPIO、定时器或其他特定于硬件的中断源。这个函数的作用是将指定的中断请求线连接到系统级中断控制器(通常是NVIC),使其能够接收到中断事件并将其传递给相关的中断处理函数。
该函数的一般调用格式如下:
```c
nrf_status_t nrf_drv_common_irq_enable(uint32_t irq_num);
```
其中,`irq_num` 参数是中断编号,对应具体的硬件中断请求,例如 `GPIOTE_IRQn` 或 `TIMER0_IRQn` 等。
在使用这个函数之前,通常需要确保中断请求已经被正确配置和初始化,包括但不限于设置中断触发模式、优先级以及配置中断处理函数。当函数返回 `NRF_SUCCESS` 表示成功启用中断,如果失败则会返回错误码。
例如启用某个中断前的配置:
```c
// 初始化中断配置
NRF_APP_ERROR_CHECK(NRF_COMMON_APP_irq_enable(GPIOTE_IRQn)); // 启动GPIO中断
// ...其他中断配置...
// 确保中断服务注册
APP_IRQ_SERVICE_DEFINE(MyCustom_IRQn, ..., MyCustom_IRQHandler, NULL, MY_CUSTOM_IRQn, true);
// 具体中断启用
APP_IRQ_ENABLE(MyCustom_IRQn);
```
阅读全文
相关推荐
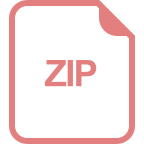
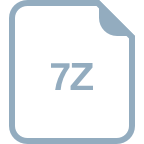
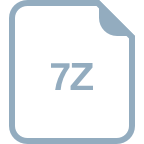
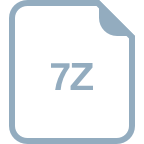
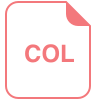











