QMatrix4x4转成 GLfloat
时间: 2023-11-03 22:05:35 浏览: 177
QMatrix4x4是Qt中的矩阵类,GLfloat是OpenGL中的浮点型数组。将QMatrix4x4转换为GLfloat数组可以通过以下代码实现:
```c++
QMatrix4x4 matrix;
GLfloat m[16];
const float* data = matrix.constData();
for (int i = 0; i < 16; i++) {
m[i] = data[i];
}
```
其中,`constData()`函数返回矩阵的数据指针,`m`数组存储了转换后的数据。转换后的数组中,每4个元素代表矩阵的一行。
相关问题
qt 将三维点阵练成曲线
在Qt中,可以使用QOpenGLFunctions和QOpenGLShader类来绘制三维图形,并将三维点阵练成曲线。以下是一个简单的例子,演示如何使用QOpenGLFunctions和QOpenGLShader类绘制三维曲线:
1. 首先,在Qt的工程文件(.pro)中添加以下代码:
```
QT += opengl
```
2. 创建一个继承自QOpenGLWidget的OpenGL窗口类,并实现以下虚函数:
```
class GLWidget : public QOpenGLWidget, protected QOpenGLFunctions
{
public:
GLWidget(QWidget *parent = nullptr);
~GLWidget();
protected:
void initializeGL() override;
void resizeGL(int w, int h) override;
void paintGL() override;
};
```
3. 在initializeGL函数中,初始化OpenGL相关参数,创建并编译shader:
```
void GLWidget::initializeGL()
{
initializeOpenGLFunctions();
// 设置背景颜色
glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
// 创建shader程序
QOpenGLShaderProgram program;
program.addShaderFromSourceCode(QOpenGLShader::Vertex,
"attribute vec3 vertex;\n"
"uniform mat4 projectionMatrix;\n"
"uniform mat4 modelViewMatrix;\n"
"void main() {\n"
" gl_Position = projectionMatrix * modelViewMatrix * vec4(vertex, 1.0);\n"
"}\n");
program.addShaderFromSourceCode(QOpenGLShader::Fragment,
"void main() {\n"
" gl_FragColor = vec4(1.0, 1.0, 1.0, 1.0);\n"
"}\n");
program.link();
program.bind();
}
```
在上面的代码中,我们首先调用initializeOpenGLFunctions函数初始化OpenGL函数,然后设置背景颜色为黑色。接着,我们创建了一个shader程序,并使用addShaderFromSourceCode函数向程序中添加了顶点着色器和片段着色器的代码。顶点着色器用来对顶点进行变换,将三维点阵转换为二维屏幕坐标;片段着色器用来对片段进行着色,我们这里将所有片段都设置为白色。最后,我们调用link和bind函数将shader程序绑定到OpenGL上下文中。
4. 在paintGL函数中,绘制三维曲线:
```
void GLWidget::paintGL()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// 设置模型视图矩阵和投影矩阵
QMatrix4x4 modelViewMatrix;
modelViewMatrix.setToIdentity();
modelViewMatrix.translate(0.0f, 0.0f, -10.0f);
modelViewMatrix.rotate(45.0f, 1.0f, 1.0f, 1.0f);
QMatrix4x4 projectionMatrix;
projectionMatrix.setToIdentity();
projectionMatrix.perspective(60.0f, GLfloat(width()) / height(), 0.1f, 100.0f);
// 设置shader中的变量
QOpenGLShaderProgram *program = QOpenGLContext::currentContext()->functions()->glUseProgram(0);
program->setUniformValue("projectionMatrix", projectionMatrix);
program->setUniformValue("modelViewMatrix", modelViewMatrix);
// 定义三维点阵
QVector<QVector3D> points;
points << QVector3D(-1.0f, -1.0f, 0.0f)
<< QVector3D(-0.5f, 0.0f, 0.5f)
<< QVector3D(0.5f, 0.0f, -0.5f)
<< QVector3D(1.0f, 1.0f, 0.0f);
// 绘制曲线
glEnable(GL_LINE_SMOOTH);
glEnable(GL_BLEND);
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
glEnableVertexAttribArray(0);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 0, points.constData());
glDrawArrays(GL_LINE_STRIP, 0, points.size());
glDisableVertexAttribArray(0);
}
```
在上面的代码中,我们首先调用glClear函数清除颜色缓冲区和深度缓冲区。然后,我们定义了模型视图矩阵和投影矩阵,将模型视图矩阵平移到屏幕中心并绕着(1,1,1)旋转45度,将投影矩阵设置为透视投影。接着,我们设置shader中的变量,将投影矩阵和模型视图矩阵传递给顶点着色器。然后,我们定义了一个三维点阵,并使用glVertexAttribPointer函数将点阵传递给OpenGL。最后,我们启用抗锯齿和混合,并使用glDrawArrays函数绘制三维曲线。
希望这个例子能帮助你理解如何在Qt中将三维点阵练成曲线。
阅读全文
相关推荐
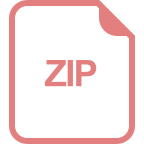
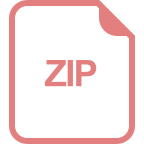
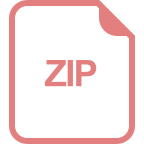
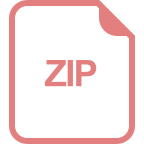
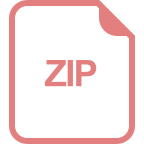
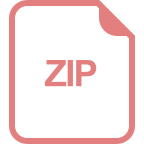