c多线程串口通信收发
时间: 2023-07-25 18:13:57 浏览: 331
实现多线程串口通信收发的关键在于使用线程来分别处理数据的发送和接收。下面是一个简单的示例代码,用于在C语言中实现多线程串口通信收发:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include <pthread.h>
int fd;
pthread_t tid[2];
char buf[256];
void *readThread(void *arg)
{
int n;
while (1) {
n = read(fd, buf, sizeof(buf));
if (n > 0) {
buf[n] = '\0';
printf("Received: %s", buf);
}
}
return NULL;
}
void *writeThread(void *arg)
{
char input[256];
while (1) {
fgets(input, sizeof(input), stdin);
write(fd, input, sizeof(input));
}
return NULL;
}
int main(int argc, char **argv)
{
struct termios tio;
fd = open(argv[1], O_RDWR | O_NOCTTY);
tcgetattr(fd, &tio);
cfsetospeed(&tio, B9600);
cfsetispeed(&tio, B9600);
tio.c_cflag &= ~PARENB;
tio.c_cflag &= ~CSTOPB;
tio.c_cflag &= ~CSIZE;
tio.c_cflag |= CS8;
tio.c_cflag &= ~CRTSCTS;
tio.c_cflag |= CREAD | CLOCAL;
tio.c_iflag &= ~(IXON | IXOFF | IXANY);
tio.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
tio.c_oflag &= ~OPOST;
tio.c_cc[VMIN] = 1;
tio.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &tio);
pthread_create(&tid[0], NULL, readThread, NULL);
pthread_create(&tid[1], NULL, writeThread, NULL);
pthread_join(tid[0], NULL);
pthread_join(tid[1], NULL);
return 0;
}
```
在这个示例代码中,我们使用了两个线程,一个用于读取串口数据,另一个用于向串口发送数据。在 `main` 函数中,我们首先打开了串口设备文件,并对其进行了初始化设置,然后创建了两个线程分别处理数据的发送和接收。最后,我们通过调用 `pthread_join` 函数等待线程的结束。
需要注意的是,由于涉及到串口的操作,因此在使用这个示例代码时需要根据实际情况修改串口的配置参数和设备文件名。
阅读全文
相关推荐






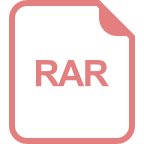







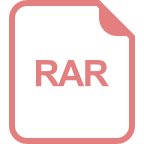
