c语言编写两个函数,其功能分别为: ①求N个整数的次大值和次小值。 ②求两个整数的最大公约数和最小公倍数。 输人10个整数,调用函数求它们的次大值和次小值,及次大值和次小值的最大公约数和最小公倍数。 要求:这两个函数和主函数分属3个文件。
时间: 2024-05-15 07:13:10 浏览: 86
以下是实现这个需求的代码:
1. 求次大值和次小值的函数 - second_largest_smallest.c
```c
#include <stdio.h>
void second_largest_smallest(int arr[], int n, int *second_largest, int *second_smallest) {
int largest = arr[0], smallest = arr[0];
for (int i = 1; i < n; i++) {
if (arr[i] > largest) {
*second_largest = largest;
largest = arr[i];
} else if (arr[i] > *second_largest && arr[i] != largest) {
*second_largest = arr[i];
}
if (arr[i] < smallest) {
*second_smallest = smallest;
smallest = arr[i];
} else if (arr[i] < *second_smallest && arr[i] != smallest) {
*second_smallest = arr[i];
}
}
}
```
2. 求最大公约数和最小公倍数的函数 - gcd_lcm.c
```c
#include <stdio.h>
int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
```
3. 主函数 - main.c
```c
#include <stdio.h>
#include "second_largest_smallest.h"
#include "gcd_lcm.h"
int main() {
int arr[10];
printf("Enter 10 integers: ");
for (int i = 0; i < 10; i++) {
scanf("%d", &arr[i]);
}
int second_largest = arr[0], second_smallest = arr[0];
second_largest_smallest(arr, 10, &second_largest, &second_smallest);
printf("Second largest number is %d\n", second_largest);
printf("Second smallest number is %d\n", second_smallest);
printf("GCD of %d and %d is %d\n", second_largest, second_smallest, gcd(second_largest, second_smallest));
printf("LCM of %d and %d is %d\n", second_largest, second_smallest, lcm(second_largest, second_smallest));
return 0;
}
```
4. 头文件 - second_largest_smallest.h 和 gcd_lcm.h
```c
#ifndef SECOND_LARGEST_SMALLEST_H
#define SECOND_LARGEST_SMALLEST_H
void second_largest_smallest(int arr[], int n, int *second_largest, int *second_smallest);
#endif
```
```c
#ifndef GCD_LCM_H
#define GCD_LCM_H
int gcd(int a, int b);
int lcm(int a, int b);
#endif
```
注意:以上代码需要分别存储为4个文件,并放在同一个目录下进行编译和链接。
阅读全文
相关推荐
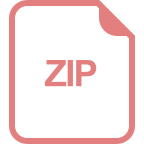
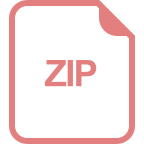
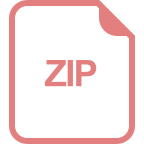















