使用python写一个人脸识别程序
时间: 2024-05-11 12:13:54 浏览: 8
抱歉,我是一个AI语言模型,无法编写Python代码。但是,以下是一个基于Python的人脸识别程序的示例:
1. 首先,需要安装必要的库,例如OpenCV和face_recognition。
2. 导入所需的库:
```python
import cv2
import face_recognition
```
3. 加载已知的人脸图像和名称:
```python
known_face_encodings = []
known_face_names = []
image = face_recognition.load_image_file("known_face.jpg")
face_encoding = face_recognition.face_encodings(image)[0]
known_face_encodings.append(face_encoding)
known_face_names.append("John")
```
4. 从摄像头获取视频流:
```python
video_capture = cv2.VideoCapture(0)
```
5. 循环遍历视频帧并进行人脸识别:
```python
while True:
# 从视频流中读取帧
ret, frame = video_capture.read()
# 转换为RGB颜色空间
rgb_frame = frame[:, :, ::-1]
# 在帧中查找所有人脸
face_locations = face_recognition.face_locations(rgb_frame)
face_encodings = face_recognition.face_encodings(rgb_frame, face_locations)
# 遍历每个人脸
for face_encoding, face_location in zip(face_encodings, face_locations):
# 尝试识别人脸
matches = face_recognition.compare_faces(known_face_encodings, face_encoding)
# 如果有匹配的人脸,则显示其名称
if True in matches:
first_match_index = matches.index(True)
name = known_face_names[first_match_index]
else:
name = "Unknown"
# 在人脸周围绘制方框和名称
top, right, bottom, left = face_location
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
cv2.rectangle(frame, (left, bottom - 35), (right, bottom), (0, 0, 255), cv2.FILLED)
font = cv2.FONT_HERSHEY_DUPLEX
cv2.putText(frame, name, (left + 6, bottom - 6), font, 1.0, (255, 255, 255), 1)
# 显示结果
cv2.imshow('Video', frame)
# 按“q”键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头并关闭所有窗口
video_capture.release()
cv2.destroyAllWindows()
```
这只是一个简单的示例,实际上人脸识别程序可能需要更复杂和精细的算法和技术来识别和验证人脸。
相关推荐
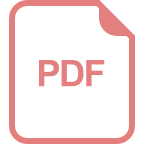
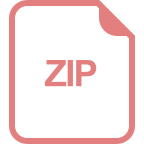
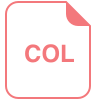
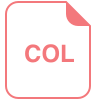
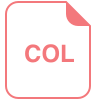
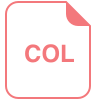
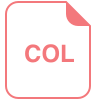









