递归计算二叉树的深度和叶子结点的个数算法分析
时间: 2023-06-25 08:02:10 浏览: 60
计算二叉树的深度和叶子节点个数,可以使用递归算法。首先,对于叶子节点,其深度为1,叶子节点数为1;对于非叶子节点,其深度为左右子树深度的最大值加1,叶子节点数为左右子树叶子节点数之和。
具体算法如下:
1. 如果二叉树为空,则深度为0,叶子节点为0。
2. 如果二叉树只有一个根节点,则深度为1,叶子节点为1。
3. 如果二叉树有左子树,递归计算左子树的深度和叶子节点个数。
4. 如果二叉树有右子树,递归计算右子树的深度和叶子节点个数。
5. 对于非叶子节点,深度为左右子树深度的最大值加1,叶子节点数为左右子树叶子节点数之和。
6. 返回深度和叶子节点数。
以下是 Python 代码实现:
```
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def depthAndLeafCount(root):
if root is None:
return 0, 0
if root.left is None and root.right is None:
return 1, 1
left_depth, left_leaf_count = depthAndLeafCount(root.left)
right_depth, right_leaf_count = depthAndLeafCount(root.right)
depth = max(left_depth, right_depth) + 1
leaf_count = left_leaf_count + right_leaf_count
return depth, leaf_count
```
其中depthAndLeafCount函数返回一个元组,第一个元素为二叉树的深度,第二个元素为二叉树的叶子节点数。
相关推荐
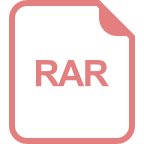














