递归计算二叉树的深度和叶子结点的个数
时间: 2023-06-25 12:02:12 浏览: 45
计算二叉树的深度可以通过递归实现。对于一个二叉树,其深度等于其左右子树深度的较大值加1。当遍历到空节点时,返回0。
计算叶子节点的个数也可以通过递归实现。对于一个节点,如果它是叶子节点,则返回1,否则返回左右子树叶子节点个数之和。
下面是代码实现,假设二叉树节点的定义如下:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
```
计算深度和叶子节点个数的函数分别为 `get_depth` 和 `get_leaf_count`:
```python
def get_depth(root: TreeNode) -> int:
if not root:
return 0
left_depth = get_depth(root.left)
right_depth = get_depth(root.right)
return max(left_depth, right_depth) + 1
def get_leaf_count(root: TreeNode) -> int:
if not root:
return 0
if not root.left and not root.right:
return 1
return get_leaf_count(root.left) + get_leaf_count(root.right)
```
我们可以构造一个二叉树进行测试:
```
1
/ \
2 3
/ \
4 5
```
```python
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.right.left = TreeNode(4)
root.right.right = TreeNode(5)
print(get_depth(root)) # 输出 3
print(get_leaf_count(root)) # 输出 3
```
相关推荐
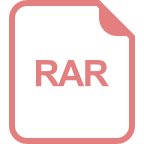














