统计二叉树中叶子结点的个数,计算二叉树的深度
时间: 2023-11-13 07:07:53 浏览: 37
计算二叉树中叶子节点的个数:
可以通过递归方式完成:
```
int count_leaf(TreeNode* root) {
if (root == nullptr) return 0;
if (root->left == nullptr && root->right == nullptr) return 1;
return count_leaf(root->left) + count_leaf(root->right);
}
```
计算二叉树的深度:
同样可以通过递归方式完成:
```
int count_depth(TreeNode* root) {
if (root == nullptr) return 0;
return max(count_depth(root->left), count_depth(root->right)) + 1;
}
```
注意,以上代码仅供参考,具体实现可能需要根据实际情况进行修改。
相关问题
计算二叉树中叶子结点的个数
计算二叉树中叶子节点的个数可以通过递归实现。具体实现方法如下:
1. 如果当前节点为空,则返回0。
2. 如果当前节点为叶子节点,则输出该节点的值并返回1。
3. 如果当前节点不是叶子节点,则递归计算左子树和右子树的叶子节点个数,并将它们相加返回。
在Java中,可以定义一个TestTree类,其中包含一个testTree方法,该方法接收一个Test类型的参数,表示二叉树的根节点。具体实现代码如下:
```
class TestTree{
public int testTree(Test test){
if(test==null){
return 0;
}
if(test.left==null&&test.right==null){
System.out.println("叶子节点:"+test.val);
return 1;
}
return testTree(test.left)+testTree(test.right);
}
}
```
同时,还需要定义一个Test类,表示二叉树的节点。该类包含一个整型变量val,以及左右
计算二叉树中叶子结点的个数 java
计算二叉树中叶子节点的个数可以通过递归实现。具体实现方法如下:
1. 如果当前节点为空,则返回0。
2. 如果当前节点为叶子节点,则输出该节点的值并返回1。
3. 如果当前节点不是叶子节点,则递归计算左子树和右子树的叶子节点个数,并将它们相加返回。
在Java中,可以定义一个TestTree类,其中包含一个testTree方法,该方法接收一个Test类型的参数,表示二叉树的根节点。具体实现代码如下:
```
class TestTree{
public int testTree(Test test){
if(test==null){
return 0;
}
if(test.left==null&&test.right==null){
System.out.println("叶子节点:"+test.val);
return 1;
}
return testTree(test.left)+testTree(test.right);
}
}
```
同时,还需要定义一个Test类,表示二叉树的节点。该类包含一个整型变量val,以及左右子节点left和right。在Test类中,还可以定义一个main方法,用于创建二叉树并计算叶子节点的个数。具体实现代码如下:
```
public class Test {
int val;
Test left;
Test right;
public Test(int val) {
this.val = val;
}
public static void main(String[] args) {
Test test = new Test(9);
test.left=new Test(5);
test.right=new Test(13);
test.left.left=new Test(3);
test.left.right=new Test(8);
test.right.left=new Test(11);
test.right.right=new Test(15);
TestTree tree =new TestTree();
System.out.println("叶子节点个数:"+tree.testTree(test));
}
}
```
运行上述代码,可以得到输出结果为:
```
叶子节点:3
叶子节点:8
叶子节点:11
叶子节点:15
叶子节点个数:4
```
相关推荐
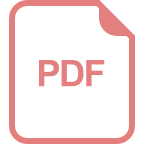












