(1)创建二叉树 (2)求二叉树的前序遍历序列 (3)求二叉树的中序遍历序列 (4)求二叉树的后序遍历序列 (5)求二叉树的层次遍历序列 (6)计算二叉树的深度 (7)统计二叉树中叶子结点的个数 (8)判断两棵树是否相等 (9)交换二叉树中每个结点的左孩子和右孩子 (10)复制二叉树
时间: 2023-06-21 11:12:59 浏览: 71
1. 创建二叉树
二叉树是一种树形结构,每个节点最多有两个子节点。我们可以通过递归的方式来创建二叉树。假设我们有一个数组arr表示二叉树的结构,-1表示空节点,其他数字表示节点的值。我们可以采用如下方式来创建二叉树:
```python
class Node:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def create_tree(arr, index):
if index >= len(arr) or arr[index] == -1:
return None
root = Node(arr[index])
root.left = create_tree(arr, 2*index+1)
root.right = create_tree(arr, 2*index+2)
return root
```
其中create_tree函数是递归创建二叉树的函数,参数arr表示数组,index表示当前节点在数组中的下标。如果当前节点为-1或者下标超出数组范围,则返回None,否则就创建一个节点,并将左子树和右子树递归创建。
2. 求二叉树的前序遍历序列
前序遍历是指先遍历根节点,然后遍历左子树,最后遍历右子树。我们可以采用递归的方式来实现前序遍历。
```python
def preorder_traversal(root):
if not root:
return []
res = [root.val]
res += preorder_traversal(root.left)
res += preorder_traversal(root.right)
return res
```
其中preorder_traversal函数是递归实现前序遍历的函数,参数root表示根节点。如果根节点为空,则返回空列表。否则就先将根节点的值加入结果列表,然后递归遍历左子树和右子树,并将结果合并。
3. 求二叉树的中序遍历序列
中序遍历是指先遍历左子树,然后遍历根节点,最后遍历右子树。我们可以采用递归的方式来实现中序遍历。
```python
def inorder_traversal(root):
if not root:
return []
res = inorder_traversal(root.left)
res += [root.val]
res += inorder_traversal(root.right)
return res
```
其中inorder_traversal函数是递归实现中序遍历的函数,参数root表示根节点。如果根节点为空,则返回空列表。否则就先递归遍历左子树,然后将根节点的值加入结果列表,最后递归遍历右子树,并将结果合并。
4. 求二叉树的后序遍历序列
后序遍历是指先遍历左子树,然后遍历右子树,最后遍历根节点。我们可以采用递归的方式来实现后序遍历。
```python
def postorder_traversal(root):
if not root:
return []
res = postorder_traversal(root.left)
res += postorder_traversal(root.right)
res += [root.val]
return res
```
其中postorder_traversal函数是递归实现后序遍历的函数,参数root表示根节点。如果根节点为空,则返回空列表。否则就先递归遍历左子树和右子树,然后将根节点的值加入结果列表,并将结果合并。
5. 求二叉树的层次遍历序列
层次遍历是指按照树的层次遍历节点。我们可以采用队列来实现二叉树的层次遍历。
```python
def level_order_traversal(root):
if not root:
return []
res = []
queue = [root]
while queue:
cur_level = []
for i in range(len(queue)):
node = queue.pop(0)
cur_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
res.append(cur_level)
return res
```
其中level_order_traversal函数是实现二叉树层次遍历的函数,参数root表示根节点。如果根节点为空,则返回空列表。否则就先将根节点加入队列,然后依次将队列中的节点取出来,并将它们的左子树和右子树加入队列,直到队列为空。
6. 计算二叉树的深度
二叉树的深度是指从根节点到叶子节点的最长路径长度。我们可以采用递归的方式来计算二叉树的深度。
```python
def tree_depth(root):
if not root:
return 0
left_depth = tree_depth(root.left)
right_depth = tree_depth(root.right)
return max(left_depth, right_depth) + 1
```
其中tree_depth函数是递归实现计算二叉树深度的函数,参数root表示根节点。如果根节点为空,则返回0。否则就递归计算左子树和右子树的深度,并取最大值加1。
7. 统计二叉树中叶子结点的个数
叶子节点是指没有子节点的节点。我们可以采用递归的方式来统计二叉树中叶子节点的个数。
```python
def count_leaf_node(root):
if not root:
return 0
if not root.left and not root.right:
return 1
return count_leaf_node(root.left) + count_leaf_node(root.right)
```
其中count_leaf_node函数是递归实现统计叶子节点个数的函数,参数root表示根节点。如果根节点为空,则返回0。否则就判断当前节点是否为叶子节点,如果是则返回1,否则递归计算左子树和右子树的叶子节点个数,并将它们相加。
8. 判断两棵树是否相等
判断两棵树是否相等,需要对两棵树进行遍历,并比较每个节点的值是否相等。我们可以采用递归的方式来判断两棵树是否相等。
```python
def is_same_tree(p, q):
if not p and not q:
return True
if not p or not q:
return False
if p.val != q.val:
return False
return is_same_tree(p.left, q.left) and is_same_tree(p.right, q.right)
```
其中is_same_tree函数是递归实现判断两棵树是否相等的函数,参数p和q分别表示两棵树的根节点。如果两棵树的根节点都为空,则返回True;如果其中有一棵树的根节点为空,则返回False;否则就比较两棵树的根节点的值是否相等,然后递归比较左子树和右子树。
9. 交换二叉树中每个结点的左孩子和右孩子
交换二叉树中每个节点的左孩子和右孩子,可以采用递归的方式来实现。
```python
def invert_tree(root):
if not root:
return None
root.left, root.right = root.right, root.left
invert_tree(root.left)
invert_tree(root.right)
return root
```
其中invert_tree函数是递归实现交换二叉树中每个节点的左孩子和右孩子的函数,参数root表示根节点。如果根节点为空,则返回None;否则就先交换根节点的左孩子和右孩子,然后递归交换左子树和右子树。
10. 复制二叉树
复制一棵二叉树,可以采用递归的方式来实现。
```python
def copy_tree(root):
if not root:
return None
new_root = Node(root.val)
new_root.left = copy_tree(root.left)
new_root.right = copy_tree(root.right)
return new_root
```
其中copy_tree函数是递归实现复制二叉树的函数,参数root表示根节点。如果根节点为空,则返回None;否则就先创建一个新节点并赋值为原节点的值,然后递归复制左子树和右子树,并将它们赋值给新节点的左孩子和右孩子。最后返回新根节点。
相关推荐
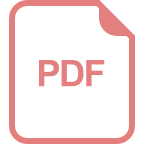
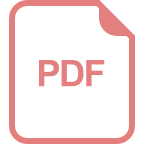















