请使用二叉树的先序遍历算法,统计二叉树中叶子结点的个数(假设二叉树已经使用链式存储;根结点的指t为Btree *root)。不需要函数模板。 函数原型:void leaf_count(Btree *root,int &k) 其中指针root指向二叉树的根结点,参数k为统计叶子结点数。
时间: 2023-12-03 20:44:54 浏览: 29
下面是使用二叉树的先序遍历算法,统计二叉树中叶子结点的个数的代码:
```c++
struct Btree{
int data;
Btree *lchild;
Btree *rchild;
};
void leaf_count(Btree *root, int &k){
if(root == nullptr){
return;
}
if(root->lchild == nullptr && root->rchild == nullptr){
k++;
}
leaf_count(root->lchild, k);
leaf_count(root->rchild, k);
}
```
在上面的代码中,我们首先判断根节点是否为叶子结点,如果是,则将叶子结点的个数加1。然后递归遍历左子树和右子树,统计每个子树中的叶子结点数量。
调用该函数的代码如下:
```c++
int main(){
Btree *root = new Btree{1, nullptr, nullptr};
root->lchild = new Btree{2, nullptr, nullptr};
root->rchild = new Btree{3, nullptr, nullptr};
root->lchild->lchild = new Btree{4, nullptr, nullptr};
root->lchild->rchild = new Btree{5, nullptr, nullptr};
root->rchild->lchild = new Btree{6, nullptr, nullptr};
root->rchild->rchild = new Btree{7, nullptr, nullptr};
int k = 0;
leaf_count(root, k);
cout << "叶子结点的个数为:" << k << endl;
return 0;
}
```
输出结果为:
```
叶子结点的个数为:4
```
其中二叉树的结构如下:
```
1
/ \
2 3
/ \ / \
4 5 6 7
```
相关推荐
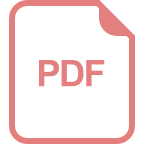
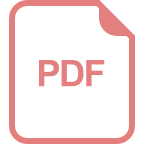
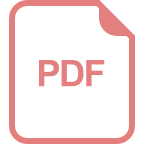














