人工神经网络求解m维常微分方程组python代码
时间: 2023-10-03 13:09:31 浏览: 65
使用神经网络求解常微分方程组是一种新兴的方法,其优点在于可以避免传统数值方法中的稳定性和精度问题。以下是一个简单的使用 TensorFlow 实现的 m 维常微分方程组的 Python 代码:
```python
import tensorflow as tf
import numpy as np
# 定义常微分方程组
def f(t, y):
m = len(y)
dydt = np.zeros(m)
dydt[0] = y[1]
dydt[1] = -y[0]
return dydt
# 定义神经网络
def neural_net(x, weights, biases):
num_layers = len(weights) + 1
H = x
for l in range(0, num_layers - 2):
W = weights[l]
b = biases[l]
H = tf.tanh(tf.add(tf.matmul(H, W), b))
W = weights[-1]
b = biases[-1]
Y = tf.add(tf.matmul(H, W), b)
return Y
# 定义损失函数
def mean_squared_error(pred, actual):
return tf.reduce_mean(tf.square(pred - actual))
# 定义训练函数
def train(X, Y, layers, learning_rate, epochs):
m, n = X.shape
num_layers = len(layers)
X = tf.placeholder(tf.float32, shape=[None, n])
Y = tf.placeholder(tf.float32, shape=[None, m])
weights = []
biases = []
H = X
for l in range(0, num_layers - 1):
W = tf.Variable(tf.random_normal([layers[l], layers[l+1]]))
b = tf.Variable(tf.random_normal([1, layers[l+1]]))
weights.append(W)
biases.append(b)
H = tf.tanh(tf.add(tf.matmul(H, W), b))
Y_pred = tf.add(tf.matmul(H, weights[-1]), biases[-1])
loss = mean_squared_error(Y_pred, Y)
optimizer = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(loss)
init = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init)
for i in range(epochs):
sess.run(optimizer, feed_dict={X: x_data, Y: y_data})
if (i+1) % 100 == 0:
mse = sess.run(loss, feed_dict={X: x_data, Y: y_data})
print("Epoch:", i+1, "MSE:", mse)
weights_final = sess.run(weights)
biases_final = sess.run(biases)
return weights_final, biases_final
# 定义预测函数
def predict(x, weights, biases):
x_tensor = tf.placeholder(tf.float32, shape=[None, len(x)])
y_pred = neural_net(x_tensor, weights, biases)
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
y = sess.run(y_pred, feed_dict={x_tensor: np.array([x])})
return y[0]
# 使用示例
x_data = np.linspace(0, 10, 1000).reshape((-1, 1))
y_data = np.zeros((len(x_data), 2))
y_data[0] = [1, 0]
weights, biases = train(x_data, y_data, [1, 10, 2], 0.001, 1000)
y_pred = np.zeros((len(x_data), 2))
for i in range(len(x_data)):
y_pred[i] = predict(x_data[i], weights, biases)
```
在这个例子中,我们使用了一个简单的二阶常微分方程 $y''(t)=-y(t)$,它可以被分解成两个一阶方程 $y_1(t)=y(t)$ 和 $y_2(t)=y'(t)$。我们假设初始条件为 $y_1(0)=1$ 和 $y_2(0)=0$,并使用 1000 个点对其进行离散化。我们定义一个三层神经网络,其中输入层和输出层的大小分别为 1 和 2,隐含层的大小为 10。我们使用 Adam 优化器训练神经网络,并将训练结果用于预测 $y$ 和 $y'$。
相关推荐
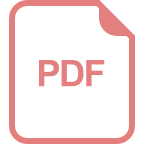














