解释以下代码# -*- coding: UTF-8 -*- import numpy as np import queue import prettytable as pt def find_zero(num): tmp_x, tmp_y = np.where(num == 0) return tmp_x[0], tmp_y[0] def swap(num_data, direction): x, y = find_zero(num_data) num = np.copy(num_data) if direction == 'left': if y == 0: # print('不能左移') return num num[x][y] = num[x][y - 1] num[x][y - 1] = 0 return num if direction == 'right': if y == 2: # print('不能右移') return num num[x][y] = num[x][y + 1] num[x][y + 1] = 0 return num if direction == 'up': if x == 0: # print('不能上移') return num num[x][y] = num[x - 1][y] num[x - 1][y] = 0 return num if direction == 'down': if x == 2: # print('不能下移') return num else: num[x][y] = num[x + 1][y] num[x + 1][y] = 0 return num def cal_wcost(num): # return sum(sum(num != end_data)) - int(num[1][1] != 0) con = 0 for i in range(3): for j in range(3): tmp_num = num[i][j] compare_num = end_data[i][j] if tmp_num != 0: con += tmp_num != compare_num return con def data_to_int(num): value = 0 for i in num: for j in i: value = value * 10 + j return value def sorte_by_floss(): tmp_open = opened.queue.copy() length = len(tmp_open) for i in range(length): for j in range(length): if tmp_open[i].f_loss < tmp_open[j].f_loss: tmp = tmp_open[i] tmp_open[i] = tmp_open[j] tmp_open[j] = tmp if tmp_open[i].f_loss == tmp_open[j].f_loss: if tmp_open[i].step > tmp_open[j].step: tmp = tmp_open[i] tmp_open[i] = tmp_open[j] tmp_open[j] = tmp opened.queue = tmp_open
时间: 2023-06-17 11:02:22 浏览: 117
这段代码实现了一个八数码问题的解法,其中包括以下函数:
- `find_zero(num)`:用于找到给定数组中数值为0的位置。
- `swap(num_data, direction)`:用于将给定数组中的0与它相邻的数进行交换。
- `cal_wcost(num)`:用于计算当前数组与目标数组之间的差异程度(估价函数)。
- `data_to_int(num)`:将给定数组中的所有数值按照顺序组合成一个整数。
- `sorte_by_floss()`:将已经扩展的节点按照f值进行排序。
这些函数都是八数码问题解法中的基础操作,可以用于构建A*算法等解法。
相关问题
# -*- coding: UTF-8 -*- import numpy as np import queue import prettytable as pt def find_zero(num): tmp_x, tmp_y = np.where(num == 0) return tmp_x[0], tmp_y[0] def swap(num_data, direction): x, y = find_zero(num_data) num = np.copy(num
_data) if direction == 'up': num[x][y], num[x-1][y] = num[x-1][y], num[x][y] elif direction == 'down': num[x][y], num[x+1][y] = num[x+1][y], num[x][y] elif direction == 'left': num[x][y], num[x][y-1] = num[x][y-1], num[x][y] elif direction == 'right': num[x][y], num[x][y+1] = num[x][y+1], num[x][y] return num def is_valid(num): if np.array_equal(num, [[1,2,3],[4,5,6],[7,8,0]]): return True else: return False def bfs(start): q = queue.Queue() q.put(start) visited = set() visited.add(start.tobytes()) while not q.empty(): curr = q.get() if is_valid(curr): return curr for direction in ['up', 'down', 'left', 'right']: new_state = swap(curr, direction) if new_state.tobytes() not in visited: visited.add(new_state.tobytes()) q.put(new_state) def main(): start_state = np.array([[2,8,3],[1,6,4],[7,0,5]]) end_state = np.array([[1,2,3],[4,5,6],[7,8,0]]) print('Start state:') tb_start = pt.PrettyTable() tb_start.add_rows(start_state) print(tb_start) print('End state:') tb_end = pt.PrettyTable() tb_end.add_rows(end_state) print(tb_end) result = bfs(start_state) print('Solution:') tb_result = pt.PrettyTable() tb_result.add_rows(result) print(tb_result) if __name__ == '__main__': main()
这是一个使用 BFS 算法解决八数码问题的 Python 代码。算法的主要思路是从起始状态开始,每次尝试将数字 0 上下左右移动,生成新的状态。然后判断这个状态是否是目标状态,如果是,就返回。如果不是,就将这个状态加入队列,并标记为已访问过。接着继续从队列中取出下一个状态,重复上述过程,直到找到目标状态或者队列为空为止。
代码中使用了 NumPy 库来处理矩阵,使用了 Queue 库来实现队列。使用了 PrettyTable 库来美化输出结果。
# -*- coding: UTF-8 -*- # -*- coding: UTF-8 -*- import math def IsPrime(v): if v<1: return 'NO' for i in range(2,v): #**********begin1********** if v%i______0: #**********end1********** return 'NO
以下是代码的完整版:
```
# -*- coding: UTF-8 -*-
import math
def IsPrime(v):
if v < 1:
return 'NO'
for i in range(2, int(math.sqrt(v)) + 1):
if v % i == 0:
return 'NO'
return 'YES'
```
这段代码定义了一个 `IsPrime` 函数,用于判断一个数是否为质数。其中,`v` 为待判断的数。如果 `v` 小于 1,直接返回 `'NO'`。否则,从 2 开始遍历到 `v` 的平方根(向上取整),如果存在任意一个数可以整除 `v`,说明 `v` 不是质数,返回 `'NO'`,否则返回 `'YES'`。这里使用了 `math` 模块的 `sqrt` 函数来计算平方根。
阅读全文
相关推荐
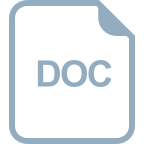
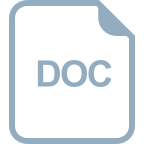
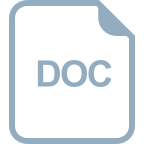




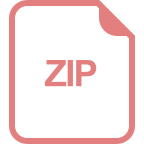









