用turtle编写程序模拟随机行走,以坐标(0, 0)为中心来绘制20X20的方格,从中心点开始,模拟画出一条行走路线,同一个点只能走一次,只能在所画的方格内行走,画出这个行走路线,直到无路可走。无路可走包括两种情况:走到边界或者当前位置的四个方向都走过了。
时间: 2023-09-23 10:06:40 浏览: 66
### 回答1:
好的,这是一个很有趣的编程问题。以下是使用Python的turtle库来实现这个程序的示例代码:
```
import turtle
import random
# 设置方格大小和中心点坐标
grid_size = 20
center = (0, 0)
# 创建turtle对象并设置画笔属性
t = turtle.Turtle()
t.speed(0)
t.pensize(2)
# 创建一个列表来记录已经走过的点
visited = [center]
# 定义一个函数来判断某个点是否可以继续走
def can_move(point):
x, y = point
return abs(x) <= (grid_size / 2) and abs(y) <= (grid_size / 2) and (x, y) not in visited
# 定义一个函数来模拟随机行走
def random_walk():
while True:
# 判断当前点是否可以继续走
if not can_move(t.position()):
break
# 随机选择一个方向并前进一步
direction = random.choice(['north', 'south', 'east', 'west'])
if direction == 'north':
t.setheading(90)
elif direction == 'south':
t.setheading(270)
elif direction == 'east':
t.setheading(0)
elif direction == 'west':
t.setheading(180)
t.forward(grid_size)
# 记录已经走过的点
visited.append(t.position())
# 将turtle移动到中心点并开始随机行走
t.penup()
t.goto(center)
t.pendown()
random_walk()
# 隐藏turtle并等待用户关闭窗口
t.hideturtle()
turtle.done()
```
这个程序运行起来会在窗口中绘制一个20X20的方格,并从中心点开始绘制一条随机行走路径,直到无路可走为止。由于随机行走是随机的,因此每次运行程序都会得到不同的结果。
### 回答2:
可以使用turtle库来编写模拟随机行走的程序。首先,需要将turtle库导入,并创建一个Turtle对象,命名为t。
接下来,需要设置一个20x20的方格,可以使用循环来绘制20个正方形。每个正方形的边长为50,绘制到适当的位置即可。
接下来,需要定义行走的算法。首先,需要在(0, 0)位置放置小海龟(t.goto(0, 0)),然后开始行走。
行走的算法可以使用while循环来实现。首先,需要判断当前位置是否是边界位置。如果当前位置的x坐标等于-500或500,或者y坐标等于-500或500,则视为走到了边界,退出循环(break)。否则,需要判断当前位置是否所有的四个方向都走过了。可以使用一个布尔变量is_all_directions_walked来记录四个方向是否都走过了,初始值设为True。然后,根据当前位置的坐标,通过条件判断来更新is_all_directions_walked的值。如果is_all_directions_walked为True,则说明当前位置无路可走,退出循环(break)。
在循环内部,可以使用randrange函数来随机选择一个方向进行行走。假设方向为0,表示向上移动一格;方向为1,表示向右移动一格;方向为2,表示向下移动一格;方向为3,表示向左移动一格。使用tsetheading函数将小海龟设置为对应的方向,并使用t.forward(50)函数向对应方向移动一格。
最后,可以使用turtle库提供的done函数来保持程序在绘制完成后不退出。
下面是一个可能的解决方案:
```python
import turtle
import random
# 设置绘制界面
screen = turtle.Screen()
screen.setup(1100, 1100)
# 创建海龟对象
t = turtle.Turtle()
t.speed(5)
t.pensize(2)
# 画方格
for i in range(20):
t.up()
t.goto(-500, -500 + i * 50)
t.down()
t.forward(1000)
t.up()
t.goto(-500 + i * 50, -500)
t.down()
t.setheading(90)
t.forward(1000)
# 开始行走
t.up()
t.home()
t.pendown()
while True:
x, y = t.position()
# 判断是否走到边界
if x == -500 or x == 500 or y == -500 or y == 500:
break
# 判断四个方向是否都走过了
is_all_directions_walked = True
if (x+50, y) in t.undobuffer() or (x, y+50) in t.undobuffer() or (x-50, y) in t.undobuffer() or (x, y-50) in t.undobuffer():
is_all_directions_walked = False
# 无路可走
if is_all_directions_walked:
break
# 随机选择方向,0:上,1:右,2:下,3:左
direction = random.randrange(4)
if direction == 0:
t.setheading(90)
t.forward(50)
elif direction == 1:
t.setheading(0)
t.forward(50)
elif direction == 2:
t.setheading(270)
t.forward(50)
else:
t.setheading(180)
t.forward(50)
# 结束
turtle.done()
```
这个程序可以模拟随机行走,并在画布上绘制出行走的轨迹,当走到边界或所有方向都走过后会停止。
### 回答3:
可以使用turtle库来编写程序,实现随机行走的模拟。具体步骤如下:
1. 导入turtle库并初始化画笔。
2. 设定方格的大小为20,以坐标(0, 0)为中心绘制20x20的方格。
3. 设置画笔的起始位置为中心点((0, 0))。
4. 创建一个列表visited,用于记录已经访问过的坐标点。
5. 循环直到无路可走:
- 随机选择一个方向(上、下、左、右)。
- 根据选择的方向移动画笔。
- 检查移动后的位置是否在方格内并且未被访问过,若满足条件,将该位置加入visited列表。
- 如果当前位置的四个方向都已经访问过或者当前位置是边界,说明无路可走,跳出循环。
6. 结束绘画。
代码示例:
```python
import turtle
import random
# 设置画布大小
canvas_size = 400
turtle.setup(canvas_size+50, canvas_size+50)
# 初始化画笔
screen = turtle.Screen()
screen.bgcolor("white")
pen = turtle.Turtle()
pen.speed(10)
# 设置方格尺寸
grid_size = 20
# 绘制方格
def draw_grid():
for i in range(-canvas_size//2, canvas_size//2+1, grid_size):
pen.penup()
pen.goto(i, -canvas_size//2)
pen.pendown()
pen.goto(i, canvas_size//2)
pen.penup()
pen.goto(-canvas_size//2, i)
pen.pendown()
pen.goto(canvas_size//2, i)
# 获得随机方向
def get_random_direction():
direction = random.choice(['UP', 'DOWN', 'LEFT', 'RIGHT'])
return direction
# 随机行走
def random_walk():
visited = [(0, 0)]
pen.penup()
pen.goto(0, 0)
pen.pendown()
while True:
direction = get_random_direction()
if direction == 'UP':
pen.setheading(90)
pen.forward(grid_size)
elif direction == 'DOWN':
pen.setheading(-90)
pen.forward(grid_size)
elif direction == 'LEFT':
pen.setheading(180)
pen.forward(grid_size)
else:
pen.setheading(0)
pen.forward(grid_size)
new_position = (pen.xcor(), pen.ycor())
if (abs(new_position[0]) > canvas_size//2-grid_size/2 or
abs(new_position[1]) > canvas_size//2-grid_size/2 or
new_position in visited):
break
visited.append(new_position)
# 绘制方格
draw_grid()
# 随机行走
random_walk()
# 结束绘画
turtle.done()
```
运行以上代码,即可通过turtle库模拟随机行走的过程,并在绘制的20x20方格内画出行走路线,直到无路可走。
相关推荐
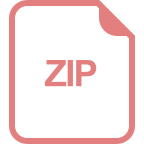
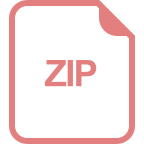














