write a program to search an element in an array
时间: 2024-09-12 10:17:02 浏览: 52
在Python中,你可以编写一个函数来搜索数组(列表)中的特定元素。这里有一个简单的线性搜索算法的例子:
```python
def search_element(arr, target):
"""
在给定的数组中查找目标元素
:param arr: 输入的数组
:param target: 要查找的目标元素
:return: 如果找到元素,返回其索引;如果没有找到,返回-1
"""
for index, element in enumerate(arr): # 使用enumerate获取元素及其索引
if element == target: # 检查当前元素是否等于目标
return index # 找到就直接返回索引
return -1 # 遍历结束后未找到,返回-1表示目标不存在
# 示例数组
array = [4, 2, 7, 1, 5]
target = 7
# 调用函数
result = search_element(array, target)
if result != -1:
print(f"元素 {target} 在数组中的索引是 {result}")
else:
print(f"元素 {target} 不在数组中")
```
这个函数通过逐个比较数组中的元素和目标值,一旦找到就立即返回其索引。如果遍历完整个数组都没找到,最后返回-1。
相关问题
Binary search is a common search approach for a list of values. For binary search to work, the elements in the array must already be ordered. Assume that the array is in ascending order. The binary search first compares the key with the element in the middle of the array. Consider the following three cases: ■ If the key is less than the middle element, you need to continue to search for the key only in the first half of the array. ■ If the key is equal to the middle element, the search ends with a match. ■ If the key is greater than the middle element, you need to continue to search for the key only in the second half of the array. Implement the binary search using Language C. And write a program to test whether it can work or not.
Here is a sample implementation of binary search in C:
```
#include <stdio.h>
int binarySearch(int arr[], int left, int right, int key) {
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == key) {
return mid;
}
else if (arr[mid] < key) {
left = mid + 1;
}
else {
right = mid - 1;
}
}
return -1;
}
int main() {
int arr[] = { 2, 4, 6, 8, 10, 12, 14, 16, 18, 20 };
int n = sizeof(arr) / sizeof(arr[0]);
int key = 12;
int index = binarySearch(arr, 0, n - 1, key);
if (index == -1) {
printf("Element not found\n");
}
else {
printf("Element found at index %d\n", index);
}
return 0;
}
```
In this implementation, `binarySearch()` takes four arguments: the array to search `arr`, the left and right indices of the subarray to search `left` and `right`, and the key to search for `key`. It returns the index of the key in the array if it is found, or `-1` if it is not found.
The function works by repeatedly dividing the subarray in half using the middle index `mid`, comparing the key to the element at `arr[mid]`, and adjusting the left and right indices accordingly until the key is found or the subarray is exhausted.
In the `main()` function, we test the `binarySearch()` function by searching for the key `12` in the array `arr`. If the key is found, we print its index; otherwise, we print a message indicating that the element was not found.
Write a program to determine and display the smallest and largest valuescontained in 99-elcment floating-point array w.
Sure, here's a program in C to determine and display the smallest and largest values contained in a 99-element floating-point array `w`:
```c
#include <stdio.h>
int main() {
float w[99]; // declare the array
float smallest, largest; // variables to store the smallest and largest values
// code to fill the array with values goes here
// initialize smallest and largest to the first element of the array
smallest = w[0];
largest = w[0];
// loop through the array and update smallest and largest as necessary
for (int i = 1; i < 99; i++) {
if (w[i] < smallest) {
smallest = w[i];
}
if (w[i] > largest) {
largest = w[i];
}
}
// display the smallest and largest values
printf("Smallest value: %f\n", smallest);
printf("Largest value: %f\n", largest);
return 0;
}
```
Note that you'll need to add code to fill the array `w` with values before the loop that determines the smallest and largest values.
阅读全文
相关推荐
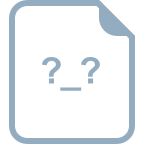
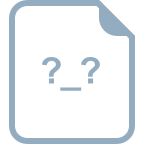
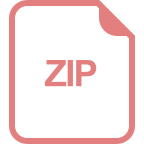













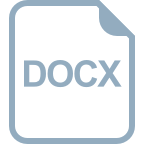