Write the following code in c #, Write a method that creates and return an array of ints. The method takes a single int argument that represents the how many items will be in the resulting array and does the following: • Declare an array of the required type • Allocate memory for the intended number of items • Using any looping structure, prompt the user for a number and then assign to each element. In your main method do the following: (1) call this method (you will need to supply an argument), assign the resulting value to at suitable variable and then (2) display this variable.
时间: 2024-02-13 21:03:23 浏览: 66
Sure, here is the code in C#:
```csharp
using System;
class Program
{
static void Main(string[] args)
{
int n = 5; // Change this to the number of items you want in the array
int[] arr = CreateIntArray(n);
Console.WriteLine("The resulting array is:");
foreach (int num in arr)
{
Console.Write(num + " ");
}
}
static int[] CreateIntArray(int n)
{
int[] arr = new int[n];
for (int i = 0; i < n; i++)
{
Console.Write("Enter a number for element " + i + ": ");
arr[i] = int.Parse(Console.ReadLine());
}
return arr;
}
}
```
Explanation:
The `CreateIntArray` method takes an `int` argument `n` which represents the number of items in the array. It first declares an array of `int` type with `n` elements. It then uses a `for` loop to prompt the user for a number for each element in the array and assigns it to the corresponding element. Finally, it returns the resulting array.
In the `Main` method, we call `CreateIntArray` with an argument of `n` and assign the resulting array to the `arr` variable. We then use a `foreach` loop to display each element in the array.
阅读全文
相关推荐
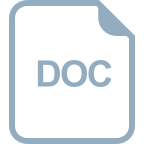
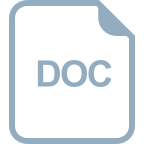
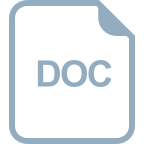














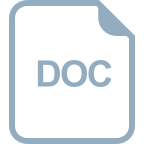
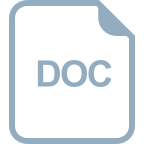