贪吃蛇游戏代码python
时间: 2024-06-11 13:02:04 浏览: 23
贪吃蛇游戏是一种经典的小游戏,它通过控制一条小蛇在屏幕上移动来吃食物,同时避免碰到墙壁或自身,直到小蛇长度达到一定程度或者游戏失败为止。下面是一个简单的Python版贪吃蛇游戏代码:
```
import pygame
import random
# 初始化游戏
pygame.init()
# 定义游戏窗口大小
width = 640
height = 480
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Snake Game")
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
# 定义蛇的初始位置和速度
snake_pos = [100, 50]
snake_body = [[100, 50], [90, 50], [80, 50]]
direction = "RIGHT"
change_to = direction
speed = 10
# 定义食物的初始位置
food_pos = [random.randrange(1, width // 10) * 10, random.randrange(1, height // 10) * 10]
food_spawn = True
# 定义分数和字体
score = 0
font_style = pygame.font.SysFont(None, 30)
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
change_to = "LEFT"
elif event.key == pygame.K_RIGHT:
change_to = "RIGHT"
elif event.key == pygame.K_UP:
change_to = "UP"
elif event.key == pygame.K_DOWN:
change_to = "DOWN"
# 判断方向是否相反
if change_to == "LEFT" and direction != "RIGHT":
direction = "LEFT"
elif change_to == "RIGHT" and direction != "LEFT":
direction = "RIGHT"
elif change_to == "UP" and direction != "DOWN":
direction = "UP"
elif change_to == "DOWN" and direction != "UP":
direction = "DOWN"
# 移动蛇的位置
if direction == "RIGHT":
snake_pos += speed
elif direction == "LEFT":
snake_pos -= speed
elif direction == "UP":
snake_pos -= speed
elif direction == "DOWN":
snake_pos += speed
# 增加蛇的长度和分数
snake_body.insert(0, list(snake_pos))
if snake_pos == food_pos:
food_spawn = False
score += 10
else:
snake_body.pop()
# 重新生成食物
if not food_spawn:
food_pos = [random.randrange(1, width // 10) * 10, random.randrange(1, height // 10) * 10]
food_spawn = True
# 绘制游戏界面
screen.fill(black)
for pos in snake_body:
pygame.draw.rect(screen, white, pygame.Rect(pos, pos, 10, 10))
pygame.draw.rect(screen, red, pygame.Rect(food_pos, food_pos, 10, 10))
# 显示分数和更新窗口
score_font = font_style.render("Score: " + str(score), True, white)
screen.blit(score_font, [5, 5])
pygame.display.update()
# 判断游戏是否结束
if snake_pos < 0 or snake_pos > width - 10 or snake_pos < 0 or snake_pos > height - 10:
pygame.quit()
quit()
for block in snake_body[1:]:
if snake_pos == block:
pygame.quit()
quit()
```
相关推荐
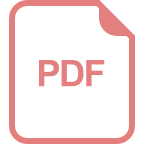













