写一个MapReduce用来将1511544070这种数据类型转变为2017-12-03这种数据类型
时间: 2024-09-18 20:05:16 浏览: 31
要实现一个简单的MapReduce程序来转换日期,我们可以假设输入的数据是一串Unix时间戳(1511544070),我们需要一个映射阶段将这些时间戳转换成标准日期格式,然后在归约阶段合并并保持唯一结果。这里是一个简化版的Java示例,使用Hadoop的InputFormat, Mapper, Reducer以及OutputFormat:
```java
// 假设我们的Mapper类
public static class DateMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
long timestamp = key.get();
String date = convertTimestampToDate(timestamp); // 自定义方法转换时间戳
context.write(new Text(date), new IntWritable(1)); // 发送键值对到reduce
}
private String convertTimestampToDate(long timestamp) {
Calendar calendar = Calendar.getInstance();
calendar.setTimeInMillis(timestamp * 1000); // Unix时间戳单位是秒,需转换毫秒
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
return sdf.format(calendar.getTime());
}
}
// 假设我们的Reducer类
public static class DateReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
@Override
protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int count = 0;
for (IntWritable value : values) {
count += value.get();
}
if (count > 0) { // 如果某个日期被映射多次,则只保留一个
context.write(key, new IntWritable(count));
}
}
}
```
在这个例子中,`DateMapper`负责接收输入的时间戳并转换为日期,然后在键上写入日期。`DateReducer`则在接收到相同日期的所有计数后,汇总并只保留最后的计数值。
请注意,这只是一个简化的示例,实际生产环境中可能还需要考虑更复杂的错误处理和输入/输出格式设置。此外,如果你正在使用Python Hadoop Streaming或者其他MapReduce框架,语法会有所不同,但基本逻辑相似。
阅读全文
相关推荐
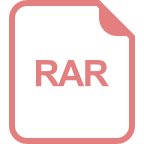
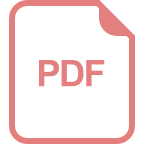
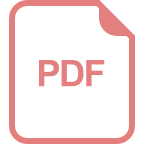
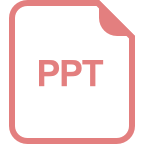
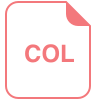
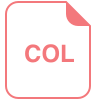
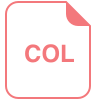
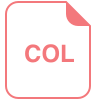
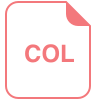
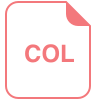
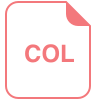
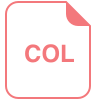
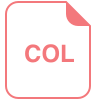
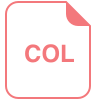
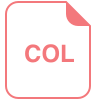
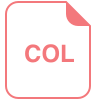