编写Java程序实现多项式乘法,多项式带余除法和多项式扩展欧几里得算法
时间: 2024-12-25 10:30:53 浏览: 9
在Java中,我们可以使用数组或列表(List)来表示多项式的系数。下面是简要的步骤来实现多项式乘法、带余除法和扩展欧几里得算法:
### 1. 多项式乘法
- 创建两个多项式的系数数组或列表。
- 初始化一个新的结果数组或列表,长度为第一个多项式的系数个数与第二个多项式的系数个数之和减一(因为会产生相同的次数项)。
- 对于每个结果的项(从0次到最高次数),遍历两个输入多项式的系数,将它们对应次幂相乘的结果累加到结果的相应位置。
```java
public List<Integer> multiplyPolynomials(List<Integer>[] polys) {
int maxDegree = polys[0].size() + polys.size() - 1;
List<Integer> result = new ArrayList<>(maxDegree + 1);
for (int i = 0; i <= maxDegree; i++) {
int sum = 0;
for (int j = 0, k = i; j < polys.size(); j++, k--) {
if (k >= polys[1].size()) {
break;
}
sum += polys.get(j) * polys.get(k);
}
result.add(sum);
}
return result;
}
```
### 2. 多项式带余除法 (除以x-a)
- 首先,你需要定义一个辅助方法来执行单次除法,即计算 `(a * b)` 的系数。
- 使用循环将多项式 `poly` 相当于 `x^n * poly(x/a)` 的形式进行迭代,直到 `poly` 变成常数项。
```java
public List<Integer> divide PolynomialByXMinusA(List<Integer> poly, int a) {
List<Integer> quotient = new ArrayList<>();
while (!poly.isEmpty()) {
int leadingTerm = poly.get(0);
int divisorTerm = a;
// 减去最大的可能商
quotient.add(leadingTerm / divisorTerm);
poly.remove(0);
// 更新多项式
for (int i = 0; i < poly.size(); i++) {
poly.set(i, poly.get(i) - leadingTerm % divisorTerm * Math.pow(a, i));
}
}
return quotient;
}
```
### 3. 多项式扩展欧几里得算法
- 这是用来找到两个多项式的最大公约数(GCD)及其贝祖等式系数,用于因式分解。
- 它涉及两步:递归地应用常规欧几里得算法得到 GCD,然后用扩展公式求解贝祖等式。
```java
public Pair<List<Integer>, List<Integer>> extendedEuclideanAlgorithm(List<Integer> poly1, List<Integer> poly2) {
if (poly2.isEmpty()) {
return new Pair<>(poly1, new ArrayList<>());
}
Pair<List<Integer>, List<Integer>> result = extendedEuclideanAlgorithm(poly2, poly1.subList(poly1.size() - poly2.size(), poly1.size()));
int gcd = result.getKey().get(0), x = result.getValue().get(0), y = result.getValue().get(1);
List<Integer> bezoutCoeffs = new ArrayList<>();
bezoutCoeffs.add(y);
for (int i = 1; i < poly1.size(); i++) {
bezoutCoeffs.add(x);
x = poly1.get(i) - gcd * x;
}
bezoutCoeffs.reverse();
return new Pair<>(gcd, bezoutCoeffs);
}
```
阅读全文
相关推荐
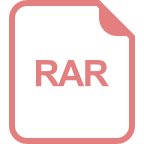
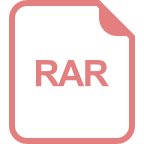
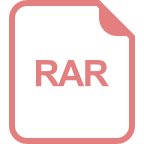

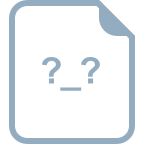
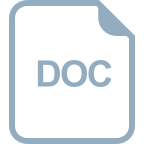
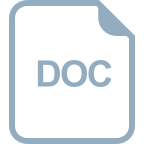
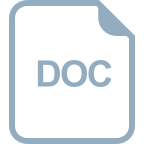
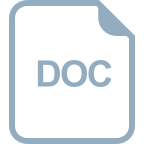








