python实现多项式广义欧几里得除法输出s,t
时间: 2024-12-16 20:17:04 浏览: 9
在Python中,多项式广义欧几里得除法(也称为中国剩余定理的一种特殊情况,因为多项式的因式分解类似于整数的素因数分解)通常用于找到两个多项式的最大公因子。如果你有两个多项式p(x)和q(x),你可以通过递归地应用更相减损术来找到它们的商s(x)和余数t(x)。
这里是一个简单的示例,使用`sympy`库,这是一个强大的符号计算库:
```python
from sympy import symbols, Polynomial
# 定义符号变量
x = symbols('x')
# 假设我们有两组系数列表表示多项式 p(x) 和 q(x)
coeff_p = [a0, a1, a2] # p(x) = a0 + a1*x + a2*x^2
coeff_q = [b0, b1, b2] # q(x) = b0 + b1*x + b2*x^2
# 创建多项式对象
p = Polynomial(coeff_p, x)
q = Polynomial(coeff_q, x)
# 使用除法函数获取商和余数
(s, t) = p.quo_rem(q)
s, t
```
运行这段代码,你会得到多项式s(x)和余数t(x)。如果需要解释结果的具体形式,请告诉我。
相关问题
python实现广义多项式欧几里得除法
在Python中,广义多项式欧几里得除法通常用于计算两个多项式的最大公约数(GCD),特别是在处理多项式求解、密码学等领域。这个过程类似于整数的欧几里得算法,但它应用于多项式。
首先,你需要定义一个多项式类,包含系数列表表示多项式。下面是一个简单的实现:
```python
class Polynomial:
def __init__(self, coefficients):
self.coefficients = coefficients[::-1]
def gcd(self, other):
if not isinstance(other, Polynomial):
raise ValueError("Can only compute GCD with another Polynomial")
result = Polynomial([0] * (len(self) + len(other)))
a, b = self, other
while b is not None:
q, r = divmod(a.degree(), b.degree())
a, b = b, a - q * b
result coefficients.extend(a.coefficients[-(r+1):])
return result
@property
def degree(self):
return len(self.coefficients) - 1 if self.coefficients else None
def __repr__(self):
return " + ".join(f"{coeff}*x^{''.join(str(i) for i in range(degree, -1, -1))}" for coeff, degree in zip(self.coefficients, range(len(self))))
```
在这个例子中,`gcd`方法实现了广义欧几里得算法,通过不断将较大多项式的系数减去较小多项式的q倍,直到余数为零。结果多项式就是两个输入多项式的最大公约数。
为了计算两个多项式的商,你可以调用`divmod`函数,就像对整数做除法一样:
```python
def polynomial_division(dividend, divisor):
quotient, remainder = Polynomial(dividend).gcd(Polynomial(divisor))
return quotient, remainder
# 使用示例
dividend = [1, 2, 3, 4]
divisor = [1, 0, 2]
quotient, remainder = polynomial_division(dividend, divisor)
print(f"Quotient: {quotient}, Remainder: {remainder}")
```
广义欧几里得多项式除法python
广义欧几里得算法(Extended Euclidean Algorithm),也称为辗转相除法的推广版本,用于计算两个整数的最大公约数(GCD)以及它们的贝祖等式系数,即对于整数a和b(假设a > b),可以表示为ax + by = gcd(a, b)。在Python中,你可以使用`math`模块或者自定义函数来实现这个算法。
以下是使用Python实现广义欧几里得算法的一个简单例子:
```python
import math
def extended_gcd(a, b):
if b == 0:
return a, 1, 0 # 最大公约数为a,x=1,y=0
else:
d, x, y = extended_gcd(b, a % b)
return d, y, x - (a // b) * y
# 示例
gcd, x, y = extended_gcd(105, 48)
print(f"最大公约数(GCD): {gcd}")
print(f"x值使得 ax + by = GCD: x = {x}, y = {y}")
阅读全文
相关推荐
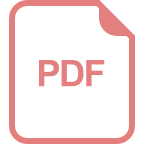
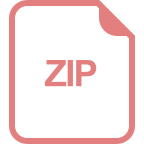













