利用c++ 做下整数的加减乘除
时间: 2024-09-29 19:08:39 浏览: 31
在C++中,你可以使用基本的算术运算符来进行整数的加减乘除操作。以下是简单的示例:
1. 加法 ( + ):
```cpp
int a = 5;
int b = 3;
int sum = a + b; // sum 现在是 8
```
2. 减法 ( - ):
```cpp
int difference = a - b; // difference 现在是 2
```
3. 乘法 ( * ):
```cpp
int product = a * b; // product 现在是 15
```
4. 除法 ( / ) 和取整除 ( / ) (注意,/ 会做地板除,结果是整数部分):
```cpp
int quotient = a / b; // quotient 现在是 1,因为有余数 2
// 或者使用 C++11 的整数除法(返回浮点数结果)
float divisionResult = static_cast<float>(a) / b; // divisionResult 约等于 1.67
```
请注意,如果除数为0,在C++中会引发异常。为了避免这种情况,你应该添加错误检查。
相关问题
C++ 最简单 计算加减乘除
在C++中,计算基本的加、减、乘、除操作是非常直观的,特别是对于整数和浮点数。以下是简单的例子:
1. **加法** (加号 `+`):
```cpp
int a = 5, b = 3;
int sum = a + b; // 等于 8
```
2. **减法** (减号 `-`):
```cpp
float c = 7.0, d = 2.5;
float difference = c - d; // 等于 4.5
```
3. **乘法** (星号 `*` 或者 `<<` 对于位运算):
```cpp
double e = 4.0;
double product = e * 2.0; // 等于 8.0
int x = 5;
x <<= 1; // 相当于 x = x * 2,即左移一位,这里是 10
```
4. **除法** (斜线 `/` 或 `>>` 对于右移,`%` 是取余运算):
```cpp
float f = 10.0;
float quotient = f / 2.0; // 等于 5.0 (向下取整)
```
请注意,以上代码片段中的除法(`/`)通常会得到浮点数结果,如果需要精确的整数除法,应该使用地板除法 (`//`)。
c++利用栈完成加减乘除及带括号的四则混合整数运算
好的,我可以为您解答这个问题。首先,您需要定义一个栈来存储中间结果和操作符。具体的做法是,读入一个整数或操作符时,如果是整数就直接入栈,如果是操作符就将其与栈顶操作符进行比较,如果优先级高于栈顶操作符,则直接入栈,否则将栈顶操作符弹出并进行相应的计算,然后将计算结果入栈。最终,栈中只剩下一个整数,即为最终的计算结果。
以下是一个示例代码:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int priority(char op) {
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
return 0;
}
int calculate(stack<int>& nums, stack<char>& ops) {
int num2 = nums.top(); nums.pop();
int num1 = nums.top(); nums.pop();
char op = ops.top(); ops.pop();
switch (op) {
case '+': return num1 + num2;
case '-': return num1 - num2;
case '*': return num1 * num2;
case '/': return num1 / num2;
default: return 0;
}
}
int evaluate(string expr) {
stack<int> nums;
stack<char> ops;
for (int i = 0; i < expr.size(); i++) {
if (isdigit(expr[i])) {
int num = 0, j = i;
while (j < expr.size() && isdigit(expr[j])) {
num = num * 10 + (expr[j] - '0');
j++;
}
nums.push(num);
i = j - 1;
} else if (expr[i] == '(') {
ops.push(expr[i]);
} else if (expr[i] == ')') {
while (ops.top() != '(') {
nums.push(calculate(nums, ops));
}
ops.pop();
} else if (expr[i] == '+' || expr[i] == '-' ||
expr[i] == '*' || expr[i] == '/') {
while (!ops.empty() && priority(ops.top()) >= priority(expr[i])) {
nums.push(calculate(nums, ops));
}
ops.push(expr[i]);
}
}
while (!ops.empty()) {
nums.push(calculate(nums, ops));
}
return nums.top();
}
int main() {
string expr = "((2+3)*5-8)/4";
int result = evaluate(expr);
cout << result << endl;
return 0;
}
```
这段代码实现了带括号的四则混合整数运算,您可以根据自己的需要进行修改。
阅读全文
相关推荐
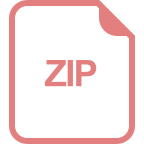
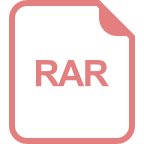













