用c++写一个程序一个矩形类,一个圆形类。一个机器人类包括多个矩形,圆形,能够通过构造函数初始化一个函数,能够通过拷贝构造函数初始化另外一个函数,能够通过wsad移动一个机器人,能够通过上下左右键移动另外一个机器人,能够输出每个机器人位置及所有参数,能够输出两个机器人之间的距离
时间: 2024-09-30 16:16:38 浏览: 27
在C++中,你可以创建一个Rectangle类和Circle类,并结合它们来构建一个Robot类。这里是一个简单的示例:
```cpp
#include <iostream>
#include <string>
class Rectangle {
public:
int x, y;
int width, height;
// 构造函数
Rectangle(int _x = 0, int _y = 0, int _width = 0, int _height = 0)
: x(_x), y(_y), width(_width), height(_height) {}
// 拷贝构造函数
Rectangle(const Rectangle &rect)
: x(rect.x), y(rect.y), width(rect.width), height(rect.height) {}
void move(int dx, int dy) {
x += dx;
y += dy;
std::cout << "Rectangle moved to (" << x << ", " << y << ")." << std::endl;
}
};
class Circle {
public:
int cx, cy;
double radius;
Circle(int _cx = 0, int _cy = 0, double _radius = 0)
: cx(_cx), cy(_cy), radius(_radius) {}
void move(int dx, int dy) {
cx += dx;
cy += dy;
std::cout << "Circle moved to (" << cx << ", " << cy << ")." << std::endl;
}
};
// Robot类
class Robot {
private:
std::vector<Rectangle> rectangles;
std::vector<Circle> circles;
public:
// 构造函数,初始化矩形和圆形
Robot(int numRects, int numCircles) {
for (int i = 0; i < numRects; ++i) {
rectangles.push_back(Rectangle());
}
for (int i = 0; i < numCircles; ++i) {
circles.push_back(Circle());
}
}
// 拷贝构造函数
Robot(const Robot &robot) {
rectangles = robot.rectangles;
circles = robot.circles;
}
// 移动操作
void moveWSAD(int w, int s, int a, int d) {
for (auto &r : rectangles) {
r.move(w, s);
}
for (auto &c : circles) {
c.move(a, d);
}
}
// 输出位置和参数
void display() const {
for (const auto &r : rectangles) {
std::cout << "Rectangle at (" << r.x << ", " << r.y << ") with size " << r.width << "x" << r.height << std::endl;
}
for (const auto &c : circles) {
std::cout << "Circle at (" << c.cx << ", " << c.cy << ") with radius " << c.radius << std::endl;
}
}
// 计算并输出两个机器人之间的欧氏距离
double distanceTo(Robot other) const {
double dist = 0;
for (size_t i = 0; i < rectangles.size(); ++i) {
dist += std::pow(other.rectangles[i].x - rectangles[i].x, 2) +
std::pow(other.rectangles[i].y - rectangles[i].y, 2);
}
for (size_t i = 0; i < circles.size(); ++i) {
dist += std::pow(other.circles[i].cx - circles[i].cx, 2) +
std::pow(other.circles[i].cy - circles[i].cy, 2);
}
return std::sqrt(dist);
}
};
```
在这个例子中,我们首先定义了Rectangle和Circle类,然后创建了一个Robot类,它持有多个矩形和圆形对象,并提供相应的移动、显示位置以及计算两者之间距离的方法。
阅读全文
相关推荐
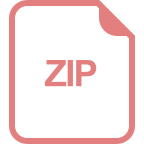
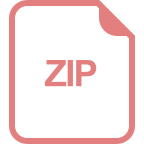
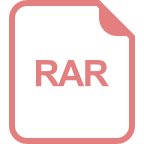



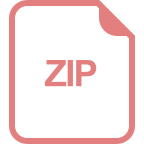
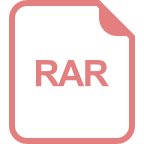
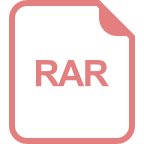
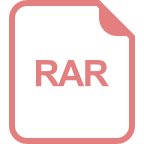
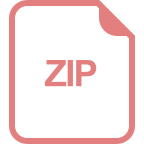
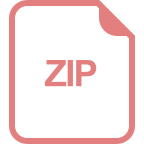
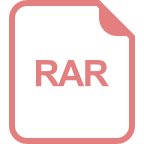
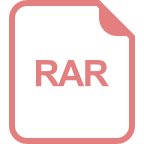
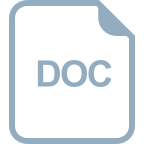




