在ros中,创建一个发布者使小海龟进行转动,当小海龟的theta为1时,停止旋转并向前运动
时间: 2024-09-27 22:07:48 浏览: 10
在Robot Operating System (ROS)中,要创建一个发布者让小海龟(通常指的是移动机器人或游戏中的模拟)根据给定的角度(如theta)进行转动和前进,你需要使用ROS的基本通信机制,即消息传递。首先,你需要定义一个包含角度信息的消息类型,比如`geometry_msgs/Twist`,这是一种常见的用于控制移动机器人的 Twist 消息。
以下是如何实现这个功能的一个简化的步骤:
1. 创建一个发布者的节点(node):
使用ROS的`std_msgs::msg::Float64`包来存储角度值,然后创建一个`geometry_msgs::msg::Twist`消息,其中`angular.z`字段表示旋转速度。
```cpp
#include <ros/ros.h>
#include <geometry_msgs/Twist.h>
class TurtlePublisher {
public:
void startPublisher() {
// 初始化 ROS 节点
ros::NodeHandle nh;
// 创建发布器,主题名为"turtle/rotate_and_move"
twist_pub_ = nh.advertise<geometry_msgs::msg::Twist>("turtle/rotate_and_move", 10); // 设置最大连接数
// 循环等待新的 theta 值
while (nh.ok()) {
double theta;
if (nh.getParam("theta", theta)) { // 获取参数
twist_.angular.z = (theta == 1.0) ? 0.0 : 1.0; // 当 theta 为 1 时停止旋转,否则继续旋转
twist_pub_.publish(twist_); // 发布 Twist 消息
}
nh.sleep(); // 等待下一帧
}
}
private:
ros::NodeHandle nh_;
ros::Publisher twist_pub_; // 发布器,用于发布 Twist 消息
geometry_msgs::msg::Twist twist_; // 存储 Twist 消息实例
};
```
2. 配置并启动发布器:
在`~/.bashrc`文件或`.bash_profile`中添加以下行以启动你的节点,并设置参数`theta`:
```bash
export ROS_MASTER_URI=http://localhost:11311
rosrun your_node_name turtle_publisher_node
rosparam set /theta 1.0 # 运行时可修改 theta 的值
```
3. 用户可以通过命令行设置`theta`参数,例如:
```
rosparam set /theta 1.5 # 将小海龟转到1.5度角后停止
```
在这个例子中,我们假设你已经有一个名为`turtle_publisher_node`的节点,你需要替换为你的实际节点名。此外,ROS master URI 应根据你的本地环境调整。如果你的小海龟还有其他特定的行为逻辑(例如前进),可以在`geometry_msgs::msg::Twist`消息中添加线速度(linear.x)。记得在`turtle_description`包中包含了小海龟的具体模型,以便于处理这些动作。
相关推荐
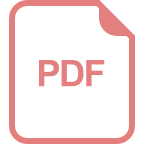
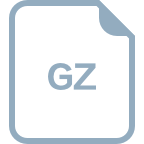
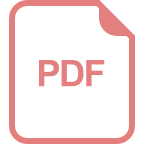













