请用rospy让ros小海龟在(5.54,5.54), (5.54,8.54), (6.54,7.54), (5.54,6.54), (5.54,5.54)这几个坐标点中循环移动,要求是先转弯后直行
时间: 2023-10-07 17:07:05 浏览: 133
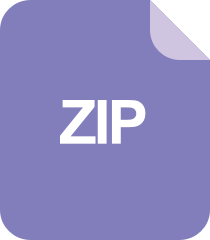
课程设计 基于MATLAB的操控ROS小海龟的上位机+GUI界面源码+GUI界面+项目资料齐全+部署说明文档.zip
以下是一个简单的示例代码,可以让ROS小海龟在指定的坐标点之间循环移动:
```python
#!/usr/bin/env python
import rospy
from geometry_msgs.msg import Twist
from turtlesim.msg import Pose
def move_turtle():
rospy.init_node('turtle_mover', anonymous=True)
velocity_publisher = rospy.Publisher('/turtle1/cmd_vel', Twist, queue_size=10)
pose_subscriber = rospy.Subscriber('/turtle1/pose', Pose, pose_callback)
# 设置目标位置列表
target_positions = [(5.54, 5.54), (5.54, 8.54), (6.54, 7.54), (5.54, 6.54), (5.54, 5.54)]
current_position = Pose()
# 等待收到当前位置
while not rospy.is_shutdown():
if current_position.x != 0.0:
break
rate = rospy.Rate(10)
# 循环移动
while not rospy.is_shutdown():
# 计算当前位置与目标位置的距离和方向
distance = ((current_position.x - target_positions[0][0])**2 + (current_position.y - target_positions[0][1])**2)**0.5
angle = atan2(target_positions[0][1] - current_position.y, target_positions[0][0] - current_position.x)
# 转向目标位置
while abs(angle - current_position.theta) > 0.1:
vel_msg = Twist()
vel_msg.angular.z = 0.5 if angle - current_position.theta > 0 else -0.5
velocity_publisher.publish(vel_msg)
rate.sleep()
angle = atan2(target_positions[0][1] - current_position.y, target_positions[0][0] - current_position.x)
# 直行到达目标位置
while distance > 0.1:
vel_msg = Twist()
vel_msg.linear.x = 0.5
velocity_publisher.publish(vel_msg)
rate.sleep()
distance = ((current_position.x - target_positions[0][0])**2 + (current_position.y - target_positions[0][1])**2)**0.5
# 到达目标位置后更新当前位置
current_position = Pose()
if len(target_positions) > 1:
target_positions = target_positions[1:]
else:
target_positions.reverse()
if __name__ == '__main__':
try:
move_turtle()
except rospy.ROSInterruptException:
pass
```
这个代码中,我们首先定义了一个`target_positions`列表,其中包含了需要移动到的坐标点。然后我们在`pose_callback`函数中订阅小海龟的位置信息,并将其保存到`current_position`变量中。
接下来,我们在`while not rospy.is_shutdown()`循环中,等待收到小海龟的位置信息。当收到位置信息后,我们开始一个`while not rospy.is_shutdown()`的循环,其中包含以下几个步骤:
1. 计算当前位置与目标位置的距离和方向。
2. 转向目标位置。
3. 直行到达目标位置。
4. 到达目标位置后更新当前位置。
在转向目标位置时,我们使用了`atan2`函数来计算当前位置与目标位置之间的角度差,并将角速度设置为0.5或-0.5,以便小海龟能够转向目标位置。
在直行到达目标位置时,我们将线速度设置为0.5,以便小海龟能够以适当的速度前进。我们在每个循环周期中使用`sleep`函数来控制小海龟的速度和频率。
在到达目标位置后,我们更新`current_position`变量,并将目标位置列表中的第一个位置弹出。如果目标位置列表为空,则将其反转,以便小海龟在完成所有坐标点后能够返回起始位置。
请注意,这只是一个简单的示例代码,您可能需要根据自己的具体需求进行修改和调整。
阅读全文
相关推荐
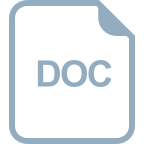
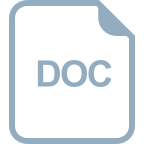









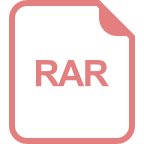
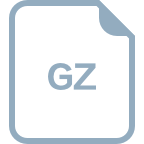
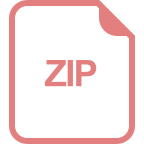
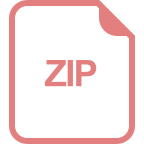


