Crop Images in Python
时间: 2024-04-09 08:25:47 浏览: 84
在Python中,可以使用多种库来裁剪图像,其中最常用的是PIL(Python Imaging Library)和OpenCV。下面是使用这两个库进行图像裁剪的示例:
使用PIL库进行图像裁剪:
```python
from PIL import Image
# 打开图像
image = Image.open("image.jpg")
# 定义裁剪区域
left = 100
top = 100
right = 300
bottom = 300
# 裁剪图像
cropped_image = image.crop((left, top, right, bottom))
# 显示裁剪后的图像
cropped_image.show()
```
使用OpenCV库进行图像裁剪:
```python
import cv2
# 读取图像
image = cv2.imread("image.jpg")
# 定义裁剪区域
x = 100
y = 100
width = 200
height = 200
# 裁剪图像
cropped_image = image[y:y+height, x:x+width]
# 显示裁剪后的图像
cv2.imshow("Cropped Image", cropped_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关问题
def load_data( *, data_dir, batch_size, image_size, class_cond=False, deterministic=False, random_crop=False, random_flip=False, ): mage.save_img(image_path, images) if not data_dir: raise ValueError("unspecified data directory") all_files = list_image_files_recursively(data_dir) # 递归的去找到所有图片文件 classes = None if class_cond: # Assume classes are the first part of the filename, # before an underscore. class_names = [bf.basename(path).split("")[0] for path in all_files] sorted_classes = {x: i for i, x in enumerate(sorted(set(class_names)))} # class id 将每一类class做成一个整型变量 classes = [sorted_classes[x] for x in class_names] dataset = ImageDataset( image_size, all_files, classes=classes, shard=MPI.COMM_WORLD.Get_rank(), num_shards=MPI.COMM_WORLD.Get_size(), random_crop=random_crop, random_flip=random_flip, ) if deterministic: loader = DataLoader( dataset, batch_size=batch_size, shuffle=False, num_workers=1, drop_last=True ) else: loader = DataLoader( dataset, batch_size=batch_size, shuffle=True, num_workers=1, drop_last=True ) while True: yield from loader,data = load_data( data_dir="F://diffuse_model_ptcg//picture//test", batch_size=4, image_size=128, class_cond=False, )for batch in data:load_data中第一个batch代表什么
在这段代码中,`load_data`函数返回一个Python生成器对象,使用`yield from loader`语句实现。每次调用这个生成器时,会从数据集中获取一个batch的数据,并将其返回。第一个batch代表的是数据集中的第一个batch,也就是在所有数据中的前`batch_size`个数据。在这个例子中,`batch_size`被设置为4,因此第一个batch代表数据集中的前4个数据。
class ImageDataset(Dataset): def init( self, resolution, image_paths, classes=None, shard=0, num_shards=1, random_crop=False, random_flip=False, ): super().init() self.resolution = resolution self.local_images = image_paths[shard:][::num_shards] self.local_classes = None if classes is None else classes[shard:][::num_shards] self.random_crop = random_crop # 随机裁剪 self.random_flip = random_flip # 随机翻转 def len(self): return len(self.local_images) # 获取数据集的数量,对于类而言,len()函数是没有办法直接计算类的长度的,如果在类中没有定义__len__()方法 # 来指明程序到底该计算哪个属性的长度时,在终端我们必须采用len(对象.属性)才能得到我们想要的结果。 def getitem(self, idx): path = self.local_images[idx] with bf.BlobFile(path, "rb") as f: pil_image = Image.open(f) pil_image.load() pil_image = pil_image.convert("RGB") if self.random_crop: arr = random_crop_arr(pil_image, self.resolution) else: arr = center_crop_arr(pil_image, self.resolution) if self.random_flip and random.random() < 0.5: arr = arr[:, ::-1] arr = arr.astype(np.float32) / 127.5 - 1 out_dict = {} if self.local_classes is not None: out_dict["y"] = np.array(self.local_classes[idx], dtype=np.int64) return np.transpose(arr, [2, 0, 1]), out_dict,如何调用其 getitem 方法
可以通过以下方式调用 `getitem` 方法:
```python
# 实例化 ImageDataset 类
dataset = ImageDataset(resolution=256, image_paths=image_paths, classes=classes, shard=0, num_shards=1, random_crop=True, random_flip=True)
# 获取第一个数据的输入和输出
x, y = dataset[0]
# 打印获取到的输入和输出
print("Input shape:", x.shape) # (3, 256, 256)
print("Output:", y) # {'y': 0},如果 ImageDataset 类中 self.local_classes 为 None,则输出为 {}
```
注意:`getitem` 方法返回的是一个元组,其中第一个元素为输入,第二个元素为输出。如果 ImageDataset 类中 self.local_classes 为 None,则输出为一个空字典。
阅读全文
相关推荐
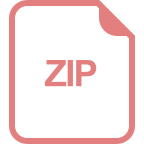
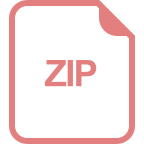
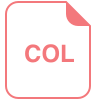
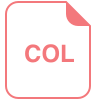
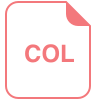
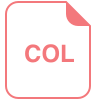
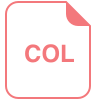
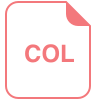





