在ubuntu环境中, (1)利用jieba库进行分词,分别统计每个文件中出现次数最多的10个词,将结果写入一个.csv文件。可自行设定是否排除单字词汇。 (2)利用wordcloud库进行词云图的绘制。
时间: 2024-12-19 19:16:06 浏览: 6
在Ubuntu环境中,你可以通过Python结合jieba库和wordcloud库来完成这个任务。以下是具体的步骤:
1. 安装必要的库:
首先需要安装`jieba`和`pandas`库用于处理文本和统计数据,以及`matplotlib`和`wordcloud`库用于绘制词云图。打开终端并运行以下命令:
```
sudo apt-get install python3-pip
pip3 install jieba pandas matplotlib wordcloud
```
2. 分词并统计词频:
使用`jieba`对每个文件的内容进行分词,并使用`pandas`库处理数据。创建一个函数来读取文件、分词和计数,然后排除单字词汇:
```python
import jieba
from collections import Counter
import pandas as pd
def process_file(file_path, exclude_single_word=True):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
words = jieba.lcut(text)
if exclude_single_word:
words = [word for word in words if len(word) > 1]
counter = Counter(words)
return counter.most_common(10)
# 对所有文件遍历并统计词频
files_to_analyze = ['file1.txt', 'file2.txt', ...] # 你需要替换为实际文件路径
all_words = [process_file(f) for f in files_to_analyze]
combined_counter = sum(all_words, [])
# 将结果保存到csv文件
df = pd.DataFrame(combined_counter, columns=['Word', 'Frequency'])
df.to_csv('top_words.csv', index=False)
```
3. 绘制词云图:
使用`wordcloud`库创建词云图,可以设置背景颜色、字体等样式。这里给出一个基本的示例,假设已经准备了统计好的词频数据:
```python
from wordcloud import WordCloud
import matplotlib.pyplot as plt
def draw_wordcloud(df, top_n=10):
most_common_words = df.head(top_n)['Word'].tolist()
wc = WordCloud(font_path='simhei.ttf', width=800, height=600).generate(" ".join(most_common_words))
plt.figure(figsize=(10, 8))
plt.imshow(wc, interpolation='bilinear')
plt.axis('off')
plt.title('Top {} Words'.format(top_n))
plt.show()
draw_wordcloud(pd.read_csv('top_words.csv'))
```
阅读全文
相关推荐
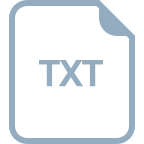
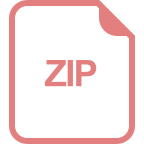
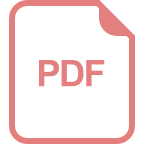















