帮我写出一段电脑游戏代码 就是单纯俄罗斯方块的小游戏代码
时间: 2023-07-10 14:14:09 浏览: 46
好的,下面是一个简单的俄罗斯方块游戏的代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 600
WINDOW_SIZE = (WINDOW_WIDTH, WINDOW_HEIGHT)
# 创建游戏窗口
screen = pygame.display.set_mode(WINDOW_SIZE)
# 设置游戏标题
pygame.display.set_caption("俄罗斯方块")
# 定义方块的大小和颜色
BLOCK_SIZE = 20
BLOCK_COLOR = (0, 255, 0)
# 定义游戏区域的大小和位置
GAME_AREA_LEFT = 50
GAME_AREA_TOP = 50
GAME_AREA_WIDTH = 10 * BLOCK_SIZE
GAME_AREA_HEIGHT = 20 * BLOCK_SIZE
# 定义游戏区域的边框颜色和宽度
GAME_AREA_BORDER_COLOR = (255, 255, 255)
GAME_AREA_BORDER_WIDTH = 2
# 定义方块的形状
BLOCK_SHAPES = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]],
[[7, 7, 7, 7]]
]
# 定义方块的类型
BLOCK_TYPES = len(BLOCK_SHAPES)
# 定义方块的初始位置
INIT_BLOCK_X = 5
INIT_BLOCK_Y = 0
# 定义方块的移动速度
BLOCK_SPEED = 1
# 定义游戏的状态
GAME_STATE_START = 0
GAME_STATE_RUN = 1
GAME_STATE_OVER = 2
# 定义游戏得分
SCORE_PER_ROW = 100
# 定义游戏的字体
FONT_SIZE = 30
FONT_COLOR = (255, 255, 255)
font = pygame.font.SysFont(None, FONT_SIZE)
# 初始化游戏状态
game_state = GAME_STATE_START
# 初始化方块的位置和形状
block_x = INIT_BLOCK_X
block_y = INIT_BLOCK_Y
block_shape = BLOCK_SHAPES[random.randint(0, BLOCK_TYPES - 1)]
# 初始化游戏区域的矩阵
game_area = [[0] * 10 for i in range(20)]
# 初始化游戏得分
score = 0
# 绘制游戏区域的边框
def draw_game_area_border():
pygame.draw.rect(screen, GAME_AREA_BORDER_COLOR, (GAME_AREA_LEFT - GAME_AREA_BORDER_WIDTH, GAME_AREA_TOP - GAME_AREA_BORDER_WIDTH, GAME_AREA_WIDTH + 2 * GAME_AREA_BORDER_WIDTH, GAME_AREA_HEIGHT + 2 * GAME_AREA_BORDER_WIDTH), GAME_AREA_BORDER_WIDTH)
# 绘制方块
def draw_block():
for i in range(len(block_shape)):
for j in range(len(block_shape[i])):
if block_shape[i][j] != 0:
pygame.draw.rect(screen, BLOCK_COLOR, (GAME_AREA_LEFT + (block_x + j) * BLOCK_SIZE, GAME_AREA_TOP + (block_y + i) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制游戏区域
def draw_game_area():
for i in range(len(game_area)):
for j in range(len(game_area[i])):
if game_area[i][j] != 0:
pygame.draw.rect(screen, BLOCK_COLOR, (GAME_AREA_LEFT + j * BLOCK_SIZE, GAME_AREA_TOP + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 判断方块是否可以移动
def can_move(x, y, shape):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
if x + j < 0 or x + j >= 10 or y + i >= 20 or (y + i >= 0 and game_area[y + i][x + j] != 0):
return False
return True
# 移动方块
def move_block(x, y, shape):
if can_move(x, y, shape):
return (x, y, shape)
else:
return (block_x, block_y, block_shape)
# 固定方块
def fix_block(x, y, shape):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
game_area[y + i][x + j] = shape[i][j]
return (INIT_BLOCK_X, INIT_BLOCK_Y, BLOCK_SHAPES[random.randint(0, BLOCK_TYPES - 1)])
# 消除行
def remove_row():
global score
rows = []
for i in range(len(game_area)):
if sum(game_area[i]) == 10:
rows.append(i)
for row in rows:
del game_area[row]
game_area.insert(0, [0] * 10)
score += SCORE_PER_ROW * len(rows)
# 绘制游戏得分
def draw_score():
score_text = font.render("Score: " + str(score), True, FONT_COLOR)
screen.blit(score_text, (10, 10))
# 绘制游戏结束界面
def draw_game_over():
game_over_text = font.render("Game Over", True, FONT_COLOR)
screen.blit(game_over_text, (WINDOW_WIDTH // 2 - FONT_SIZE * 3, WINDOW_HEIGHT // 2 - FONT_SIZE))
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if game_state == GAME_STATE_START or game_state == GAME_STATE_OVER:
if event.key == pygame.K_SPACE:
game_state = GAME_STATE_RUN
block_x, block_y, block_shape = (INIT_BLOCK_X, INIT_BLOCK_Y, BLOCK_SHAPES[random.randint(0, BLOCK_TYPES - 1)])
game_area = [[0] * 10 for i in range(20)]
score = 0
elif game_state == GAME_STATE_RUN:
if event.key == pygame.K_LEFT:
block_x -= BLOCK_SPEED
elif event.key == pygame.K_RIGHT:
block_x += BLOCK_SPEED
elif event.key == pygame.K_DOWN:
block_y += BLOCK_SPEED
elif event.key == pygame.K_UP:
block_shape = [[block_shape[j][i] for j in range(len(block_shape))] for i in range(len(block_shape[0]))]
# 游戏逻辑
if game_state == GAME_STATE_RUN:
block_x, block_y, block_shape = move_block(block_x, block_y + BLOCK_SPEED, block_shape)
if block_y == INIT_BLOCK_Y and not can_move(block_x, block_y, block_shape):
game_state = GAME_STATE_OVER
else:
block_x, block_y, block_shape = fix_block(block_x, block_y, block_shape)
remove_row()
# 绘制游戏窗口
screen.fill((0, 0, 0))
draw_game_area_border()
draw_block()
draw_game_area()
draw_score()
if game_state == GAME_STATE_OVER:
draw_game_over()
pygame.display.update()
```
这段代码使用了 Pygame 库,运行时需要先安装 Pygame 库。
相关推荐
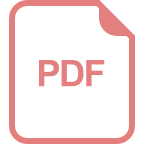
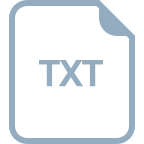
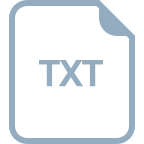
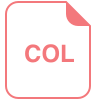
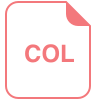
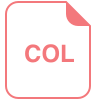
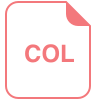
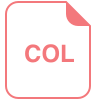









