使用torch实现图卷积神经网络
时间: 2024-03-09 22:23:30 浏览: 30
以下是使用torch实现图卷积神经网络的示例代码:
```python
import torch
import torch.nn.functional as F
from torch.nn import Linear
class GCN(torch.nn.Module):
def __init__(self, in_dim, hid_dim, out_dim):
super(GCN, self).__init__()
self.lin1 = Linear(in_dim, hid_dim)
self.lin2 = Linear(hid_dim, out_dim)
def forward(self, x, adj):
x = F.relu(self.lin1(x))
x = torch.matmul(adj, x)
x = self.lin2(x)
return x
```
在此示例中,我们定义了一个名为GCN的类,该类继承自torch.nn.Module。GCN类具有三个属性:
- in_dim:输入特征的维度。
- hid_dim:隐藏特征的维度。
- out_dim:输出特征的维度。
GCN类还有一个forward()方法,用于执行前向传递。在前向传递中,我们首先使用线性层lin1将输入特征x映射到隐藏特征h。然后,我们使用邻接矩阵adj对隐藏特征进行卷积操作。在本例中,我们使用torch.matmul()函数对邻接矩阵和隐藏特征进行矩阵乘法运算。最后,我们使用线性层lin2将卷积后的特征映射到输出特征。
下面是使用GCN类的示例代码:
```python
import torch
from GCN import GCN
# 创建模型
model = GCN(in_dim=10, hid_dim=16, out_dim=2)
# 创建输入特征和邻接矩阵
x = torch.randn(5, 10)
adj = torch.randn(5, 5)
# 执行前向传递
output = model(x, adj)
# 打印输出特征
print(output)
```
在此示例中,我们首先导入GCN类。然后,我们创建一个名为model的GCN对象,其输入特征维度为10,隐藏特征维度为16,输出特征维度为2。接下来,我们创建输入特征x和邻接矩阵adj,并将它们传递给模型的forward()方法。最后,我们打印输出特征。
相关推荐
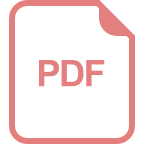
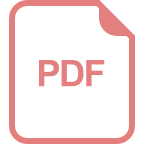
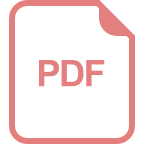














