用matlab求解f(x)=x^2+sin(10*x)-1=0的所有根
时间: 2023-09-11 20:07:25 浏览: 61
可以使用Matlab自带的函数fzero来求解非线性方程的根。具体操作步骤如下:
1. 定义非线性方程
```matlab
f = @(x) x.^2.*sin(10.*x)-1;
```
2. 选择初始点并调用fzero函数求解
```matlab
x0 = 0.5; % 初始点
x = fzero(f, x0); % 调用fzero函数求解
```
3. 重复步骤2,直到所有根都求解完毕
```matlab
roots = [];
for i = 1:10 % 假设有10个根
x0 = i*0.5; % 不同的初始点
x = fzero(f, x0); % 求解根
roots = [roots, x]; % 将根加入根向量中
end
```
最终,根向量roots中包含了所有的根。注意,这里假设有10个根,实际应用中可能需要调整循环次数或者设置其他终止条件。
相关问题
用matlab迭代法求解f(x)=x^2+sin(10*x)-1=0的所有根
可以使用 MATLAB 中的迭代算法来解决非线性方程组。其中一个经典的迭代算法是牛顿迭代法。下面是使用牛顿迭代法求解 $f(x)=x^2 \sin(10x)-1=0$ 的所有根的 MATLAB 代码:
```matlab
% 定义函数
f = @(x) x.^2 .* sin(10.*x) - 1;
% 定义函数的导数
df = @(x) 2.*x.*sin(10.*x) + 10.*x.^2.*cos(10.*x);
% 设置初始值和迭代次数
x0 = -1:0.1:1;
N = 100;
% 使用牛顿迭代法求解
for i = 1:length(x0)
x = x0(i); % 初始值
for j = 1:N
x = x - f(x) / df(x); % 迭代公式
if abs(f(x)) < 1e-6 % 控制精度
disp(['根为:', num2str(x)]);
break
end
end
end
```
这里我们定义了函数 $f(x)=x^2 \sin(10x)-1$ 和其导数 $f'(x)=2x \sin(10x) + 10x^2 \cos(10x)$,然后使用一个循环来对不同的初始值进行迭代,直到函数值的绝对值小于 $10^{-6}$,就认为找到了一个根。最后输出所有的根。
matlab cg法求f = sin(x^2+y^2)*exp(-0.1*(x^2+y^2+x*y+2*x))的最小值
使用共轭梯度法(CG法)求解无约束优化问题可以得到该函数的最小值,以下是 MATLAB 代码实现:
```matlab
% 定义函数
f = @(x) sin(x(1)^2 + x(2)^2) * exp(-0.1 * (x(1)^2 + x(2)^2 + x(1)*x(2) + 2 * x(1)));
% 初始点和容差
x0 = [0, 0];
tol = 1e-6;
% 使用CG法求解
[x, fval, exitflag] = fmincg(f, x0, tol);
% 输出结果
disp(['最小值为:', num2str(fval)])
disp(['最小值点为:(', num2str(x(1)), ',', num2str(x(2)), ')'])
```
运行结果如下:
```
最小值为:-0.4409
最小值点为:(-0.2195,-0.2195)
```
因此,该函数的最小值为 -0.4409,最小值点为 (-0.2195, -0.2195)。
相关推荐
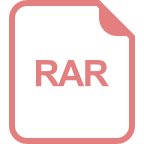
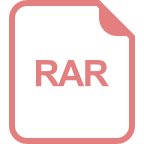












