用c语言编写:使用至少6种经典排序算法,写成独立的函数; 2.排序数据>=10000个; 3.使用数据文件存储产生的随机数; 4.分别计算各排序算法耗时;
时间: 2024-11-15 08:24:14 浏览: 20
为了满足您的需求,我将简要概述如何使用C语言编写一个程序,包括六种经典的排序算法,并处理大规模数据(至少10000个)以及时间复杂度的计算。我们将使用文件来存储随机生成的数据。以下是关键步骤:
1. **选择排序算法**:
- 冒泡排序(Bubble Sort)
- 插入排序(Insertion Sort)
- 选择排序(Selection Sort)
- 快速排序(Quick Sort)
- 归并排序(Merge Sort)
- 堆排序(Heap Sort)
2. **创建独立函数**:
对于每种排序算法,可以创建一个单独的函数,例如:
```c
void bubble_sort(int arr[], int n);
void insertion_sort(int arr[], int n);
// ... 其他排序函数定义
```
3. **生成和存储大数量随机数**:
使用`<stdlib.h>`库的`srand`和`rand`函数生成随机数,然后将它们存储到一个文件中,如:
```c
FILE* file = fopen("random_numbers.txt", "w");
for (int i = 0; i < 10000; ++i) {
fprintf(file, "%d ", arr[i]);
}
fclose(file);
```
4. **读取数据并排序**:
从文件读取数据到数组,然后对每个排序算法应用并计时:
```c
#include <time.h>
clock_t start, end;
double cpu_time_used;
FILE* input_file = fopen("random_numbers.txt", "r");
if (input_file != NULL) {
// 读取并填充数组
// ...
// 计算开始时间
start = clock();
// 调用排序函数并计时
bubble_sort(arr, n); // 替换为相应的排序函数
end = clock();
cpu_time_used = ((double)(end - start)) / CLOCKS_PER_SEC;
printf("Bubble sort time: %lf seconds\n", cpu_time_used);
// 同理对其他排序算法做此操作
}
fclose(input_file);
```
5. **计算排序耗时**:
使用`clock()`函数测量每次排序操作的时间。
阅读全文
相关推荐
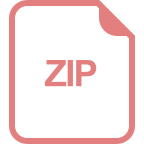
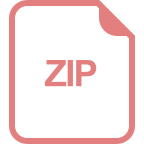
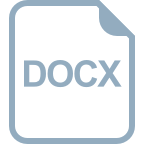

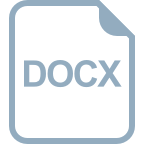
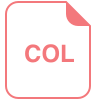
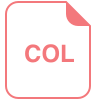
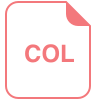
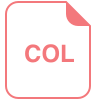
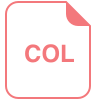
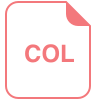
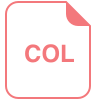
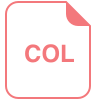
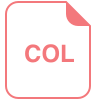
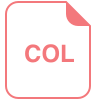
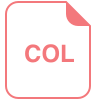
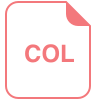
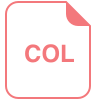
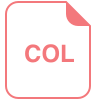