java 根据Excel导入实体stream流处理数据进行数据库操处理
时间: 2023-12-22 21:03:46 浏览: 184
可以使用Apache POI库来读取Excel文件,并使用Java 8的Stream API来处理数据,最后再进行数据库操作。下面是一个简单的示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.stream.StreamSupport;
public class ExcelImporter {
public static void main(String[] args) {
String filePath = "path/to/your/excel/file.xlsx";
try (Workbook workbook = WorkbookFactory.create(new FileInputStream(filePath))) {
Sheet sheet = workbook.getSheetAt(0); // 假设要读取第一个sheet
// 使用Stream API处理每一行的数据
StreamSupport.stream(sheet.spliterator(), false)
.skip(1) // 跳过表头行
.map(ExcelImporter::convertRowToEntity) // 将行数据转换为实体对象
.forEach(ExcelImporter::processEntity); // 处理每个实体对象,可以进行数据库操作
} catch (IOException e) {
e.printStackTrace();
}
}
private static Entity convertRowToEntity(Row row) {
// 根据Excel中的列索引获取对应数据
String col1 = row.getCell(0).getStringCellValue();
int col2 = (int) row.getCell(1).getNumericCellValue();
// 其他列...
// 创建实体对象并设置属性
Entity entity = new Entity();
entity.setCol1(col1);
entity.setCol2(col2);
// 其他属性...
return entity;
}
private static void processEntity(Entity entity) {
// 处理实体对象,可以进行数据库操作
// ...
}
}
class Entity {
private String col1;
private int col2;
// 其他属性...
// getter和setter方法...
}
```
上述代码使用Apache POI库读取Excel文件,然后使用Stream API对每一行数据进行处理,将其转换为实体对象。在`convertRowToEntity()`方法中,你可以根据Excel文件中的列索引或列名获取对应数据,并创建实体对象。在`processEntity()`方法中,你可以对每个实体对象进行处理,例如进行数据库操作。
请记得根据你的实际情况修改代码,包括Excel文件路径、sheet索引、实体类属性等。
阅读全文
相关推荐
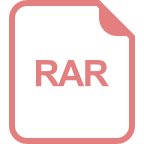
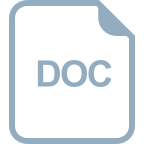
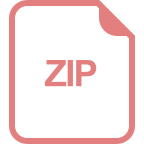
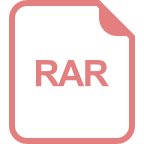
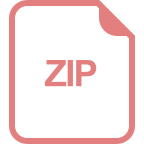
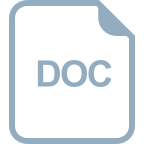
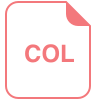
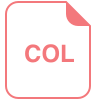
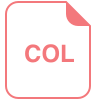
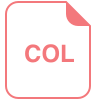
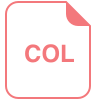

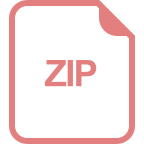
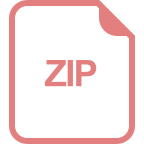
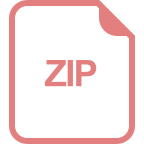
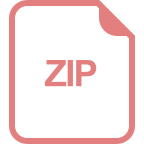