class SupPixUnpool(torch.nn.Module): def __init__(self): super(SupPixUnpool, self).__init__() def forward(self, pooled, spx): outShape = pooled.size()[0:2]+spx.size()[-2:] out = pooled.new_zeros(outShape) for batch in xrange(pooled.size()[0]): out[batch, :, :, :] = pooled[batch, :, spx[batch,:,:]] return out解释上述代码
时间: 2024-04-22 09:27:33 浏览: 10
这是一个使用PyTorch实现的超像素池化层(SupPix Pooling)的逆过程,即超像素上采样(SupPix Unpooling)的代码实现。
具体而言,这个类实现了一个名为forward的前向传播函数,该函数接受两个输入参数:一个是经过超像素池化后的tensor(pooled),另一个是对应的超像素标签(spx)。
在这个函数中,首先根据输入的pooled tensor和spx tensor计算出输出tensor的形状(outShape)。这里的输出tensor包含了pooled tensor的前两个维度(即batch size和channel数),以及spx tensor的高度和宽度。
然后,对于每个batch中的数据,通过循环遍历spx tensor中的每个超像素,将对应的pooled tensor中的值复制到输出tensor的相应位置上。
最后,返回完整的输出tensor。
相关问题
class Net(nn.Module): def __init__(self):的理解
这段代码定义了一个 PyTorch 中的神经网络模型。具体地说,它定义了一个继承自 `nn.Module` 的类 `Net`,并在该类的 `__init__` 方法中定义了模型的结构。
在 PyTorch 中,所有的神经网络模型都必须继承自 `nn.Module` 类,并在其 `__init__` 方法中定义网络的层次结构。这个 `Net` 类的 `__init__` 方法中没有接受任何参数,因此这个模型的结构是固定的,不能根据不同的输入数据进行调整。
下面是一个示例,展示了如何在 `__init__` 方法中定义一个简单的神经网络模型:
```python
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 100) # 全连接层,输入维度为 10,输出维度为 100
self.relu = nn.ReLU() # ReLU 激活函数
self.fc2 = nn.Linear(100, 1) # 全连接层,输入维度为 100,输出维度为 1
def forward(self, x):
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
return x
```
在这个例子中,我们定义了一个包含两个全连接层和一个 ReLU 激活函数的神经网络模型。在 `__init__` 方法中,我们定义了两个全连接层和一个 ReLU 激活函数,并将它们保存为类属性。这些层的参数是自动初始化的,不需要手动指定。
`forward` 方法定义了模型的前向传播过程。在这个例子中,我们按照顺序连接了两个全连接层和一个 ReLU 激活函数。`forward` 方法的输入参数 `x` 是一个张量,表示模型的输入数据。在前向传播过程中,我们将输入数据 `x` 进行一系列的线性变换和非线性变换,最终得到模型的输出结果。在这个例子中,我们的模型输出的是一个标量值。
class LinearModel(torch.nn.Module): def __init__(self): super(LinearModel, self).__init__() def forward(self, x): y_pred = self.linear(x) return y_pred
你提供的代码片段是一个简单的线性模型类的定义。它继承自PyTorch的`torch.nn.Module`类,并覆盖了`__init__`和`forward`方法。
在`__init__`方法中,你首先调用了父类的构造函数`super(LinearModel, self).__init__()`来初始化继承自`torch.nn.Module`的基类。接下来,你可以在这个方法中定义模型的结构和参数。
在`forward`方法中,你通过调用`self.linear(x)`来进行模型的前向计算。这里假设`self.linear`是一个线性层(Linear layer),它将输入`x`与权重进行线性变换,并得到预测结果`y_pred`。
注意,你提供的代码片段中没有展示出线性层的定义和初始化,你需要在`__init__`方法中添加这部分代码。例如,可以使用`self.linear = torch.nn.Linear(input_dim, output_dim)`来定义一个输入维度为`input_dim`、输出维度为`output_dim`的线性层。
最后,`forward`方法返回预测结果`y_pred`。
这个线性模型类可以用于回归问题,其中输入数据经过线性变换后得到连续的预测结果。
相关推荐
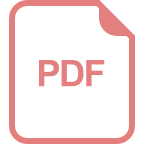
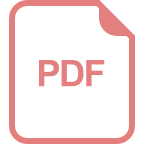
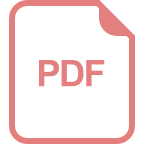













